qt最小二乘法的多项式拟合
时间: 2023-11-15 15:52:29 浏览: 78
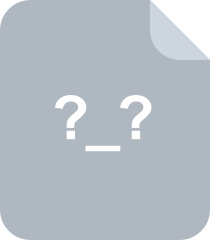
最小二乘法进行多项式拟合
在Qt中进行最小二乘法的多项式拟合,需要以下步骤:
1. 定义多项式函数
在进行多项式拟合时,需要定义一个多项式函数,例如:
```
double polyFunc(double x, QVector<double> coeffs)
{
double result = 0.0;
for (int i = 0; i < coeffs.size(); i++) {
result += coeffs[i] * qPow(x, i);
}
return result;
}
```
其中,coeffs为多项式系数,x为自变量,result为因变量。
2. 计算拟合系数
在进行拟合时,需要计算多项式系数,可以使用最小二乘法进行计算,例如:
```
QVector<double> polyfit(QVector<double> x, QVector<double> y, int order)
{
QVector<double> coeffs(order + 1);
int n = x.size();
int m = order + 1;
QVector<double> a(2 * m - 1);
QVector<double> b(m);
for (int i = 0; i < 2 * m - 1; i++) {
double sum = 0.0;
for (int j = 0; j < n; j++) {
sum += qPow(x[j], i);
}
a[i] = sum;
}
for (int i = 0; i < m; i++) {
double sum = 0.0;
for (int j = 0; j < n; j++) {
sum += y[j] * qPow(x[j], i);
}
b[i] = sum;
}
QMatrix matrix(2 * m - 1, m);
for (int i = 0; i < 2 * m - 1; i++) {
for (int j = 0; j < m; j++) {
matrix(i, j) = a[i - j + m - 1];
}
}
QVector<double> result = matrix.solve(b);
for (int i = 0; i < m; i++) {
coeffs[i] = result[i];
}
return coeffs;
}
```
其中,x和y为样本点的自变量和因变量,order为多项式的阶数,coeffs为计算出的多项式系数。
3. 绘制拟合曲线
使用得到的多项式系数,可以绘制拟合曲线,例如:
```
QVector<double> xFit;
QVector<double> yFit;
for (double x = xMin; x <= xMax; x += step) {
xFit.append(x);
yFit.append(polyFunc(x, coeffs));
}
QwtPlotCurve *curve2 = new QwtPlotCurve();
curve2->setSamples(xFit, yFit);
curve2->setPen(QPen(Qt::red));
curve2->attach(ui->qwtPlot);
```
其中,xMin和xMax为自变量的最小值和最大值,step为自变量的步长,curve2为拟合曲线的QwtPlotCurve对象。
阅读全文
相关推荐
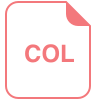
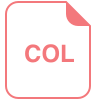
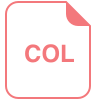
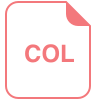
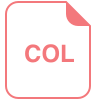
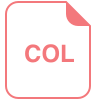
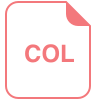
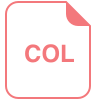


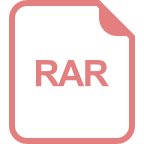
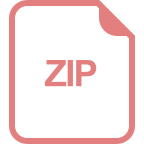
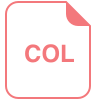
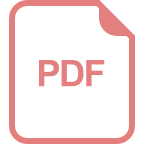
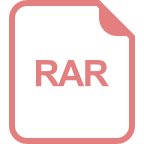