stm32按键控制呼吸灯
时间: 2023-10-21 13:04:48 浏览: 222
可以通过以下步骤实现:
1. 配置按键引脚和呼吸灯引脚的GPIO口。
2. 使用定时器来生成PWM波形控制呼吸灯亮度,可以使用TIM2或TIM3定时器,设置定时器的ARR、PSC和CCR寄存器来控制PWM波形的频率和占空比。
3. 在主函数中读取按键状态,如果按键按下,则改变PWM波形的占空比来控制呼吸灯的亮度。
下面是一个基本的示例代码:
```c
#include "stm32f10x.h"
#define LED_PIN GPIO_Pin_13
#define LED_GPIO GPIOC
#define KEY_PIN GPIO_Pin_0
#define KEY_GPIO GPIOA
void GPIO_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
/* 初始化LED引脚 */
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOC, ENABLE);
GPIO_InitStructure.GPIO_Pin = LED_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LED_GPIO, &GPIO_InitStructure);
/* 初始化按键引脚 */
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = KEY_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(KEY_GPIO, &GPIO_InitStructure);
}
void TIM_Configuration(void)
{
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
TIM_OCInitTypeDef TIM_OCInitStructure;
/* 初始化TIM2 */
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM2, ENABLE);
TIM_TimeBaseStructure.TIM_Period = 999; // PWM频率为1000Hz
TIM_TimeBaseStructure.TIM_Prescaler = 71; // TIM2时钟为72MHz,分频为72,计数频率为1MHz
TIM_TimeBaseStructure.TIM_ClockDivision = 0;
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseInit(TIM2, &TIM_TimeBaseStructure);
/* 初始化TIM2 PWM输出通道 */
TIM_OCInitStructure.TIM_OCMode = TIM_OCMode_PWM1;
TIM_OCInitStructure.TIM_OutputState = TIM_OutputState_Enable;
TIM_OCInitStructure.TIM_Pulse = 0; // 初始占空比为0
TIM_OCInitStructure.TIM_OCPolarity = TIM_OCPolarity_High;
TIM_OC1Init(TIM2, &TIM_OCInitStructure);
TIM_OC1PreloadConfig(TIM2, TIM_OCPreload_Enable);
TIM_Cmd(TIM2, ENABLE);
}
int main(void)
{
GPIO_Configuration();
TIM_Configuration();
uint8_t key_state = 0;
uint16_t pwm_duty = 0;
while (1)
{
key_state = GPIO_ReadInputDataBit(KEY_GPIO, KEY_PIN);
if (key_state == 0) // 按键按下
{
pwm_duty += 10;
if (pwm_duty > 999)
pwm_duty = 999;
TIM_SetCompare1(TIM2, pwm_duty);
}
/* 呼吸灯效果 */
for (int i = 0; i < 100; i++)
{
TIM_SetCompare1(TIM2, i);
for (int j = 0; j < 10000; j++);
}
for (int i = 100; i > 0; i--)
{
TIM_SetCompare1(TIM2, i);
for (int j = 0; j < 10000; j++);
}
}
}
```
在示例代码中,按键引脚使用了上拉输入模式,因此需要按下按键才能读取到低电平状态。呼吸灯效果采用了简单的循环控制方式,实际应用中可以根据需要选择更加复杂的控制算法。
阅读全文
相关推荐
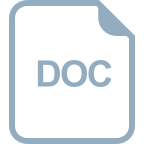
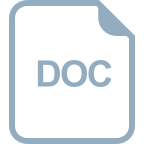
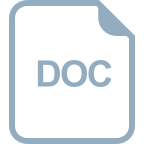






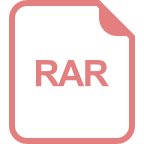
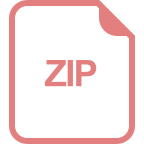
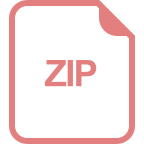






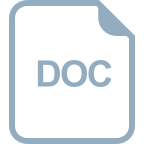