mybatis批量更新
时间: 2023-07-17 09:59:19 浏览: 62
在 MyBatis 中进行批量更新操作,可以使用 `update` 标签和 `foreach` 标签来实现。
首先,需要定义一个包含多个更新参数的列表,例如:
```
List<MyEntity> entityList = new ArrayList<MyEntity>();
entityList.add(new MyEntity(1, "value1"));
entityList.add(new MyEntity(2, "value2"));
entityList.add(new MyEntity(3, "value3"));
```
然后,在 MyBatis 的 Mapper 文件中,可以使用以下语法来实现批量更新操作:
```
<update id="batchUpdate" parameterType="java.util.List">
<foreach collection="list" item="entity" separator=";">
update my_table set my_column = #{entity.myColumn} where id = #{entity.id}
</foreach>
</update>
```
在上面的示例中,`batchUpdate` 是更新操作的 ID,`parameterType` 是传递给更新操作的参数类型,这里是一个 `java.util.List`。`foreach` 标签用于遍历更新参数列表中的每个元素,`collection` 属性指定要遍历的列表,`item` 属性指定要遍历的每个元素的变量名,`separator` 属性指定每个更新语句之间的分隔符。
在更新语句中,使用 `#{entity.myColumn}` 和 `#{entity.id}` 来引用参数列表中的每个元素的属性值。
最后,在 Java 代码中,可以调用该更新操作并传递参数列表,例如:
```
SqlSession sqlSession = sqlSessionFactory.openSession();
try {
MyMapper mapper = sqlSession.getMapper(MyMapper.class);
mapper.batchUpdate(entityList);
sqlSession.commit();
} finally {
sqlSession.close();
}
```
在这个示例中,使用 `sqlSessionFactory` 来打开一个新的 `SqlSession`,获取 `MyMapper` 接口的实现,并调用 `batchUpdate` 方法来执行批量更新操作。最后,调用 `commit` 方法提交事务,并关闭 `SqlSession`。
阅读全文
相关推荐
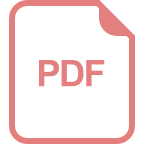
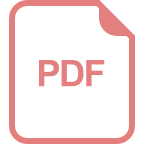
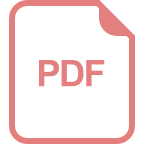








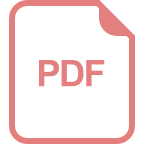
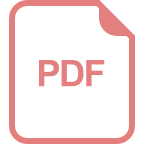
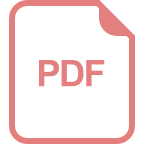
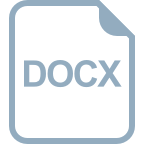
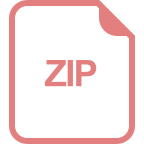
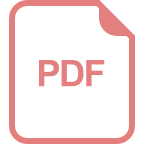