mysql中有一张表,通过id和parentid实现父子关系的关联,而且父子关系是多层.在java中如何使用mybatisplus实现.抽出某一条数据的所有父数据和子数据...
时间: 2024-05-03 18:18:26 浏览: 103
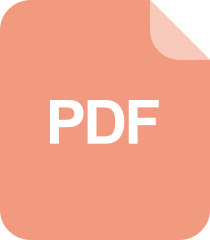
Java 将有父子关系的数据转换成树形结构数据

在Java中使用MybatisPlus实现父子关系的关联,可以使用MybatisPlus的树形结构插件TreeModel来实现。
首先,需要在实体类中定义一个父级字段和子级字段,例如:
```java
public class Entity {
private Long id;
private Long parentId;
private List<Entity> children;
// getter 和 setter 方法
}
```
然后,在Mapper接口中添加一个继承自BaseMapper的接口,并且指定实体类和主键类型:
```java
public interface EntityMapper extends BaseMapper<Entity> {
}
```
接下来,在Service层中,可以使用MybatisPlus提供的Wrapper条件构造器来实现抽出某一条数据的所有父数据和子数据,例如:
```java
@Service
public class EntityService extends ServiceImpl<EntityMapper, Entity> {
public List<Entity> getParentsAndChildren(Long id) {
List<Entity> result = new ArrayList<>();
Entity entity = this.getById(id);
if (entity != null) {
// 查询所有父数据
while (entity.getParentId() != null) {
entity = this.getById(entity.getParentId());
if (entity != null) {
result.add(entity);
}
}
// 查询所有子数据
QueryWrapper<Entity> wrapper = new QueryWrapper<>();
wrapper.eq("parent_id", id);
List<Entity> children = this.list(wrapper);
entity.setChildren(children);
result.add(entity);
}
return result;
}
}
```
在上面的代码中,通过while循环查询所有父数据,直到父级ID为null,表示已经查询到最顶层的数据。然后,使用QueryWrapper查询所有子数据,并将子数据设置到当前实体类的children字段中。最后,将当前实体类添加到结果集中返回即可。
注意:在实体类中定义的children字段必须是List类型,否则MybatisPlus的树形结构插件TreeModel无法正常工作。
阅读全文