winform datagridview 数据刷新
时间: 2024-12-27 22:15:19 浏览: 25
### 解决WinForms中DataGridView的数据刷新问题
在C# WinForms应用程序中,正确地实现`DataGridView`的数据刷新对于保持用户界面的实时性和准确性至关重要。以下是几种有效的方法来确保`DataGridView`能够及时更新显示最新的数据。
#### 方法一:重新绑定数据源
当基础数据发生变化时,可以通过再次调用`DataSource`属性并赋值新的数据集来触发视图更新。这种方法简单直接,适用于大多数场景下的批量更改情况[^1]。
```csharp
// 假设dataGrid是已经初始化好的DataGridView对象
private void RefreshData()
{
List<MyDataType> newData = GetDataFromSource(); // 获取最新数据列表
dataGrid.DataSource = null; // 清除现有绑定
dataGrid.DataSource = newData; // 绑定新数据
}
```
#### 方法二:使用BindingList<T>
通过采用`System.ComponentModel.BindingList<T>`作为数据容器,可以在不完全重建整个表格的情况下动态添加、删除或修改记录项。此类实现了INotifyPropertyChanged接口,在元素发生改变时会自动通知UI层进行相应调整[^2]。
```csharp
public class MyCustomRow : INotifyPropertyChanged
{
private string _name;
public event PropertyChangedEventHandler PropertyChanged;
protected virtual void OnPropertyChanged(string propertyName)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
public string Name
{
get => _name;
set
{
if (_name != value)
{
_name = value;
OnPropertyChanged(nameof(Name));
}
}
}
}
var bindingList = new BindingList<MyCustomRow>();
bindingList.Add(new MyCustomRow() { Name = "Item 1" });
dataGridView1.DataSource = bindingList;
```
#### 方法三:局部刷新特定行/列
如果只需要更新部分区域而非全部内容,则可以直接访问目标单元格并通过编程方式设定其值。这种方式效率较高,尤其适合处理大规模数据表中的少量变动情形[^3]。
```csharp
int rowIndexToUpdate = FindRowIndexById(5); // 找到要更新的那一行索引
if (rowIndexToUpdate >= 0 && rowIndexToUpdate < dataGridView.Rows.Count)
{
DataGridViewRow row = dataGridView.Rows[rowIndexToUpdate];
row.Cells["ColumnName"].Value = newValue;
}
```
阅读全文
相关推荐
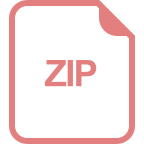
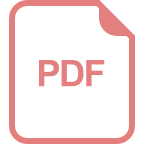
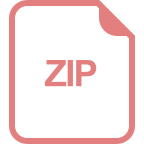

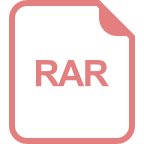
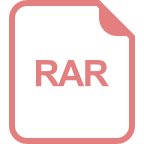
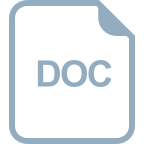
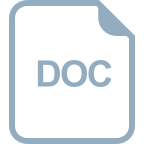
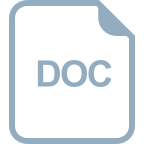




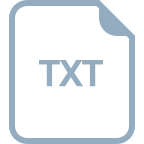



