用c++计算各类人民币张数问题
时间: 2024-09-13 16:12:32 浏览: 21
在C++中计算各类人民币张数问题,通常需要编写一个程序来处理用户的输入,并根据输入的金额计算出不同面额的人民币张数。这个问题可以通过多种方式解决,但是一个常见的方法是使用除法和取余操作来计算每种面额的张数。
以下是一个简单的示例程序,用来计算给定金额的人民币各面额张数:
```cpp
#include <iostream>
using namespace std;
void calculateMoney(int amount) {
// 定义各种面额的人民币
const int ONE_HUNDRED = 100; // 100元
const int FIFTY = 50; // 50元
const int TWENTY = 20; // 20元
const int TEN = 10; // 10元
const int FIVE = 5; // 5元
const int ONE = 1; // 1元
// 计算不同面额的张数
int hundred_count = amount / ONE_HUNDRED;
amount %= ONE_HUNDRED;
int fifty_count = amount / FIFTY;
amount %= FIFTY;
int twenty_count = amount / TWENTY;
amount %= TWENTY;
int ten_count = amount / TEN;
amount %= TEN;
int five_count = amount / FIVE;
amount %= FIVE;
int one_count = amount;
// 输出结果
cout << "100元张数:" << hundred_count << endl;
cout << "50元张数:" << fifty_count << endl;
cout << "20元张数:" << twenty_count << endl;
cout << "10元张数:" << ten_count << endl;
cout << "5元张数:" << five_count << endl;
cout << "1元张数:" << one_count << endl;
}
int main() {
int amount;
cout << "请输入金额(单位:元):";
cin >> amount;
calculateMoney(amount);
return 0;
}
```
这段代码首先定义了各个面额的人民币,并将用户的输入金额逐步除以不同面额,计算出每种面额的张数,并在最后输出结果。
相关推荐
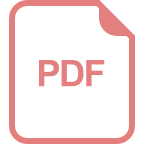
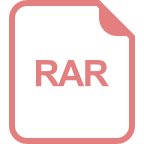
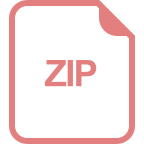














