如何实现利用 Vue 的 el-upload 组件实现文件拖拽并支持排序的功能?
时间: 2024-10-17 17:13:56 浏览: 25
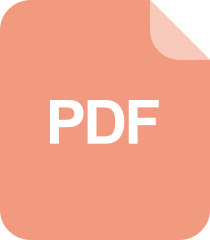
基于vue-upload-component封装一个图片上传组件的示例

在Vue中,要使用`el-upload`组件实现文件拖拽和排序功能,你可以按照以下步骤进行:
1. 引入依赖:首先,在你的Vue项目中引入Element UI库,特别是`el-upload`组件。如果还没有安装,可以运行`npm install element-ui`。
```html
<script src="https://unpkg.com/element-ui@2.15.1/lib/index.js"></script>
```
2. 安装并注册组件:在你的Vue实例中注册`ElUpload`组件。
```javascript
import { ElUpload } from 'element-ui';
Vue.component('el-upload', ElUpload);
```
3. 创建上传组件:创建一个Vue组件,设置`ref`属性获取到`el-upload`的实例,并配置必要的选项。例如,启用拖拽上传、限制一次只上传一个文件等。
```html
<template>
<div>
<el-upload
ref="upload"
:action="url"
:auto-upload="false"
drag
multiple
on-success="handleSuccess"
on-change="handleChange"
:data="fileData"
:on-drag-end="onDragEnd">
<i class="el-icon-upload"></i> 打开选择
<button slot="tip">点击或者拖拽文件</button>
</el-upload>
<!-- 拖拽排序部分 -->
<ul v-if="sortedFiles.length > 0">
<li v-for="(item, index) in sortedFiles" :key="index">
{{ item.name }}
<button @click="swap(index, index + 1)">交换</button>
</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
url: '',
fileData: {},
files: [],
sortedFiles: [],
};
},
methods: {
handleSuccess(response, file, fileList) {
// 文件上传成功处理...
},
handleChange(file) {
this.files.push(file);
if (this.sortedFiles.length === 0) {
this.sortedFiles = [...this.files];
}
},
onDragEnd(e) {
// 鼠标离开后更新排序列表
this.sortedFiles = [...this.files].sort((a, b) => a.rawIndex - b.rawIndex);
},
swap(indexA, indexB) {
const temp = this.sortedFiles[indexA];
this.sortedFiles[indexA] = this.sortedFiles[indexB];
this.sortedFiles[indexB] = temp;
},
},
};
</script>
```
在这个例子中,我们禁用了自动上传(`auto-upload`),并在`handleChange`事件中实时更新文件列表。同时,我们在`onDragEnd`事件中对文件列表进行排序,并在HTML结构中添加了交换按钮以实现文件的动态排序。
阅读全文
相关推荐
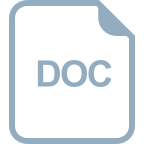
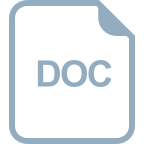


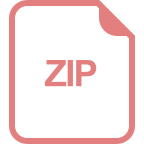
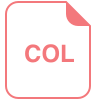
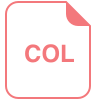
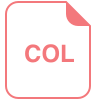
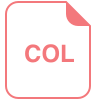
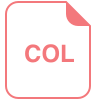







