写一个c语言程序,要求使用顺序栈和kmp算法
时间: 2024-10-11 13:07:29 浏览: 36
在C语言中,我们可以创建一个简单的顺序栈结构,并结合KMP(Knuth-Morris-Pratt)算法来处理字符串匹配。首先,我们需要定义一个顺序栈的结构体:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义顺序栈结构体
typedef struct {
char* top;
int capacity;
int size;
} Stack;
// 初始化栈
Stack* init_stack(int capacity) {
Stack* stack = (Stack*)malloc(sizeof(Stack));
stack->top = NULL;
stack->capacity = capacity;
stack->size = 0;
return stack;
}
// 入栈操作
void push(Stack* stack, char item) {
if (stack->size == stack->capacity) {
stack->capacity *= 2; // 当栈满时,容量翻倍
stack = expand_stack(stack);
}
stack->top = (char*)(stack->top + sizeof(char)); // 移动栈顶指针
*stack->top++ = item;
stack->size++;
}
// 出栈操作
char pop(Stack* stack) {
if (stack->size <= 0) {
printf("Stack is empty.\n");
return '\0';
}
char item = *stack->top - 1; // 因为移动了,所以还原位置
stack->top--;
stack->size--;
return item;
}
// 扩展栈空间
void expand_stack(Stack* stack) {
stack->top = (char*)((char*)stack->top + stack->capacity);
stack->capacity *= 2;
}
// KMP算法部分
int kmp_search(char* pattern, char* text) {
int pattern_len = strlen(pattern);
int text_len = strlen(text);
int* lps = compute_lps(pattern);
int i = 0, j = 0;
while (i < text_len && j < pattern_len) {
if (pattern[j] == text[i]) {
i++;
j++;
} else if (j > 0) {
j = lps[j - 1];
} else {
i++;
}
}
free(lps); // 释放LPS数组
return j == pattern_len ? i - j : -1; // 如果找到匹配,返回偏移量;否则返回-1
}
// 计算Pattern的最长公共前缀
int* compute_lps(char* pattern) {
int len = 0, i = 1;
int* lps = (int*)malloc(sizeof(int) * (strlen(pattern) + 1));
while (i < strlen(pattern)) {
if (pattern[i] == pattern[len]) {
len++;
lps[i] = len;
i++;
} else {
if (len != 0) {
len = lps[len - 1];
} else {
lps[i] = 0;
i++;
}
}
}
return lps;
}
int main() {
Stack* stack = init_stack(5);
// 假设我们有一个字符串列表,例如 "banana", "apples", "orange"
char words[] = {'b', 'a', 'n', 'a', 'n', 'a', ' ', 'a', 'p', 'p', 'l', 'e', 's', ' ', 'o', 'r', 'a', 'n', 'g', 'e'};
for (char word : words) {
push(stack, word);
}
char pattern[] = "ana"; // 我们想要查找的模式
int index = kmp_search(pattern, (char*)stack->top); // 使用KMP在栈顶元素中查找模式
if (index != -1) {
printf("Pattern found at position %d in the stack.\n", index);
} else {
printf("Pattern not found.\n");
}
// 清理内存
while (!isEmpty(stack)) {
pop(stack);
}
free(stack);
return 0;
}
```
这个程序首先初始化一个顺序栈,然后将一系列字符入栈。接着,它使用KMP算法在栈顶的字符串上搜索给定的模式。如果找到匹配,会打印出匹配的位置。
阅读全文
相关推荐
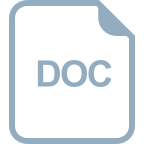
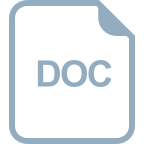
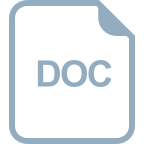
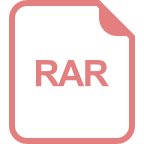
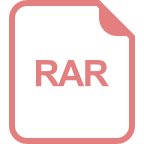
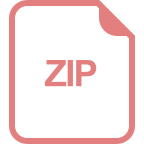
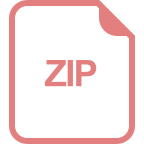
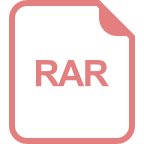
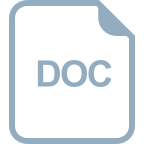
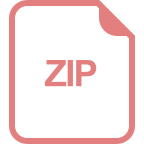
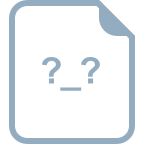
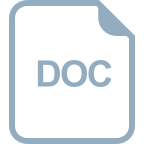
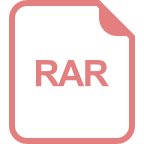
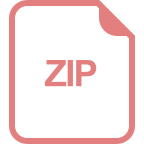
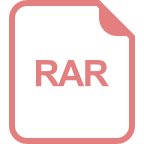
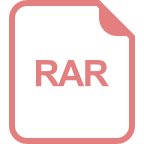
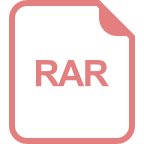
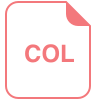
