在qt中,使用qcustomPlot绘制折线图,使用串口数据,需要实时显示并在新窗口展示,如何实现,给出代码
时间: 2024-04-13 20:29:28 浏览: 48
在Qt中使用QCustomPlot绘制折线图,并实时显示串口数据并在新窗口展示,可以按照以下步骤进行:
1. 创建一个新的Qt Widgets应用程序项目,并添加QCustomPlot库到项目中。你可以通过在.pro文件中添加以下行来包含QCustomPlot库:
```shell
LIBS += -L/path/to/qcustomplot -lqcustomplot
```
2. 在主窗口的头文件中包含以下头文件:
```cpp
#include <QMainWindow>
#include <QtSerialPort/QSerialPort>
#include "qcustomplot.h"
```
3. 在主窗口的类定义中声明QSerialPort和QCustomPlot的成员变量:
```cpp
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
private:
QSerialPort *serialPort;
QCustomPlot *customPlot;
private slots:
void readSerialData();
};
```
4. 在主窗口的构造函数中初始化QSerialPort和QCustomPlot,并连接串口数据接收的槽函数:
```cpp
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
{
serialPort = new QSerialPort(this);
customPlot = new QCustomPlot(this);
// 设置QCustomPlot的标题和轴标签等
// 连接串口数据接收的槽函数
connect(serialPort, SIGNAL(readyRead()), this, SLOT(readSerialData()));
}
```
5. 实现串口数据接收的槽函数readSerialData(),在该函数中读取串口数据并更新折线图:
```cpp
void MainWindow::readSerialData()
{
QByteArray data = serialPort->readAll();
// 处理串口数据,将数据解析为x和y坐标
// 更新折线图
customPlot->graph(0)->addData(x, y);
customPlot->xAxis->rescale();
customPlot->replot();
}
```
6. 在主窗口的析构函数中释放QSerialPort和QCustomPlot的资源:
```cpp
MainWindow::~MainWindow()
{
delete serialPort;
delete customPlot;
}
```
7. 在主函数中创建MainWindow对象并显示主窗口:
```cpp
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
MainWindow w;
w.show();
return a.exec();
}
```
以上是一个简单的示例代码,你可以根据你的实际需求进行修改和扩展。注意,你还需要在代码中设置串口的参数(如波特率、数据位、停止位等)以及打开和关闭串口的操作。
相关推荐








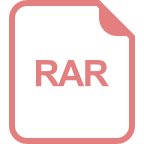
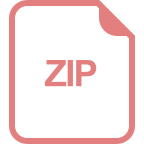
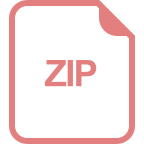