python实现物体识别的代码
时间: 2024-06-11 13:01:51 浏览: 8
Python 实现物体识别的代码,可以使用 OpenCV 或 TensorFlow 等开源库来实现。其中,OpenCV 是一个基于 BSD 许可(开源)发行的跨平台计算机视觉库,可以用来处理图像和视频等多媒体数据;TensorFlow 是一个由 Google 开发的开源机器学习框架,可以用来实现各种机器学习算法,包括物体识别。
下面是 OpenCV 和 TensorFlow 分别实现物体识别的示例代码:
OpenCV 示例代码:
```python
import cv2
# 加载分类器
cascade_path = 'haarcascade_frontalface_default.xml'
face_cascade = cv2.CascadeClassifier(cascade_path)
# 加载图像
image_path = 'test.jpg'
image = cv2.imread(image_path)
# 转换为灰度图像
gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 检测人脸
faces = face_cascade.detectMultiScale(gray_image, scaleFactor=1.1, minNeighbors=5, minSize=(30, 30))
# 绘制矩形框
for (x, y, w, h) in faces:
cv2.rectangle(image, (x, y), (x+w, y+h), (0, 255, 0), 2)
# 显示结果
cv2.imshow('result', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
TensorFlow 示例代码:
```python
import tensorflow as tf
import numpy as np
import cv2
# 加载模型
model_path = 'frozen_inference_graph.pb'
with tf.gfile.FastGFile(model_path, 'rb') as f:
graph_def = tf.GraphDef()
graph_def.ParseFromString(f.read())
# 加载标签
label_map_path = 'label_map.pbtxt'
category_index = {}
with open(label_map_path, 'r') as f:
for line in f:
if 'id:' in line:
id = int(line.split(':'))
elif 'name:' in line:
name = line.split(':').strip()[1:-1]
category_index[id] = {'name': name}
# 加载图像
image_path = 'test.jpg'
image = cv2.imread(image_path)
image_np = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
# 运行模型
with tf.Session() as sess:
tf.import_graph_def(graph_def, name='')
image_tensor = sess.graph.get_tensor_by_name('image_tensor:0')
boxes_tensor = sess.graph.get_tensor_by_name('detection_boxes:0')
scores_tensor = sess.graph.get_tensor_by_name('detection_scores:0')
classes_tensor = sess.graph.get_tensor_by_name('detection_classes:0')
num_detections_tensor = sess.graph.get_tensor_by_name('num_detections:0')
boxes, scores, classes, num_detections = sess.run([boxes_tensor, scores_tensor, classes_tensor, num_detections_tensor], feed_dict={image_tensor: np.expand_dims(image_np, axis=0)})
# 绘制矩形框和标签
for i in range(num_detections.astype(np.int32)):
if scores[0,i] > 0.5:
ymin, xmin, ymax, xmax = tuple(boxes[0,i].tolist())
(left, right, top, bottom) = (int(xmin * image.shape), int(xmax * image.shape), int(ymin * image.shape), int(ymax * image.shape))
label = category_index[int(classes[0,i])]['name']
cv2.rectangle(image, (left, top), (right, bottom), (0, 255, 0), 2)
cv2.putText(image, label, (left+10, top+20), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (255, 255, 255), 1)
# 显示结果
cv2.imshow('result', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
相关推荐
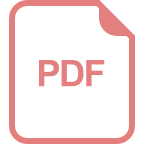














