根据文件写出响应代码、
时间: 2024-09-28 11:06:12 浏览: 32
依据您提供的实训内容,以下是各题目对应的Python代码:
### 第一题: 商店收费系统设计
```python
# 用户输入购买书籍的数量和游戏卡带的数量
book_count = int(input("请输入购买的《西游记》书本数量: "))
game_card_count = int(input("请输入购买的游戏卡带数量: "))
# 定义单价
book_price = 35
game_card_price = 120
# 根据购买数量计算折扣后的总价
total_price_books = book_count * book_price
total_price_game_cards = game_card_price * game_card_count
discount_books = 1
if book_count == 2:
discount_books = 0.9
elif book_count > 2:
discount_books = 0.8
discount_game_cards = 1 if game_card_count < 2 else 0.9
# 应付总金额
total_payment = (total_price_books * discount_books) + (total_price_game_cards * discount_game_cards)
print(f"您好,您购买了{book_count}本《西游记》,{game_card_count}个卡带,共需支付金额为:{total_payment}")
```
### 第二题: 整除判断
```python
n = int(input("请输入一个正整数N来检查其是否可以被3, 5, 或者7整除: "))
divisible_by_3 = n % 3 == 0
divisible_by_5 = n % 5 == 0
divisible_by_7 = n % 7 == 0
output_message = ""
if divisible_by_3:
output_message += "3"
if divisible_by_5:
if len(output_message) > 0:
output_message += ", "
output_message += "5"
if divisible_by_7:
if len(output_message) > 0:
output_message += ", "
output_message += "7"
if len(output_message) > 0:
print(f"{n}能被{output_message}整除")
else:
print(f"{n}不能被3,5,7整除")
```
### 第三题: 循环输出特定范围内的数字
```python
# 使用 for 循环
print("使用for循环:")
for i in range(1, 101):
if i % 3 != 0:
print(i, end=", ")
if i == 50:
print("\n", end="")
# 使用 while 循环
print("使用while循环:")
i = 1
while i <= 100:
if i % 3 != 0:
print(i, end=", ")
if i == 50:
print("\n", end="")
i += 1
```
这些脚本遵循了实训报告中的要求,并实现了预期的功能。你可以将它们复制到适当的环境中测试运行。
阅读全文
相关推荐
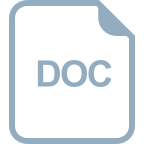
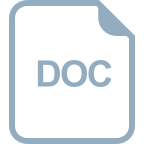
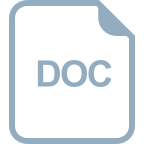
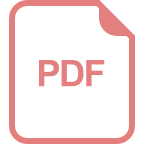
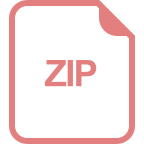
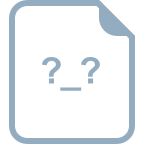
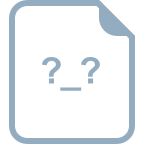
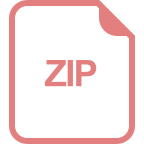
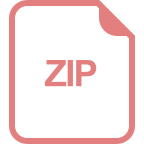
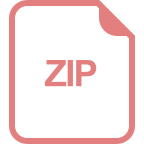
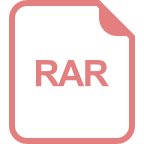
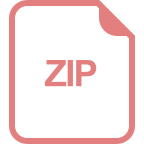
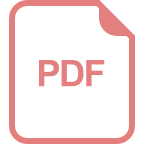
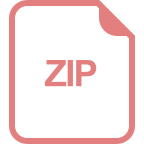
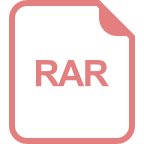
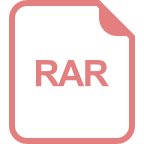
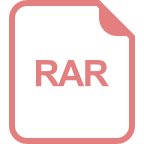
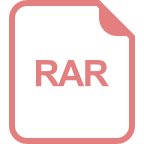
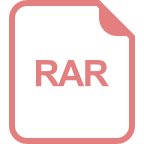