Python人脸识别ui布局代码
时间: 2024-06-27 09:00:42 浏览: 164
在Python中,使用人脸识别功能通常涉及到OpenCV库(cv2)和深度学习框架(如TensorFlow或PyTorch),而UI布局则可能用到如Tkinter、PyQt或Kivy等库。下面是一个简单的例子,展示了如何结合这些库来实现基本的人脸识别UI:
```python
import cv2
import tkinter as tk
from PIL import Image, ImageTk
# 加载预训练的人脸检测模型
face_cascade = cv2.CascadeClassifier(cv2.data.haarcascades + 'haarcascade_frontalface_default.xml')
def detect_faces(img_path):
img = cv2.imread(img_path)
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
faces = face_cascade.detectMultiScale(gray, scaleFactor=1.1, minNeighbors=5)
return faces
class FaceRecognitionApp(tk.Tk):
def __init__(self):
super().__init__()
self.label = tk.Label(self, text="Upload an image:", font=("Arial", 16))
self.label.pack(pady=10)
self.image_path = tk.StringVar()
self.file_entry = tk.Entry(self, textvariable=self.image_path)
self.file_entry.pack()
self.button = tk.Button(self, text="Detect Face", command=self.detect_face)
self.button.pack(pady=10)
def detect_face(self):
file_path = self.file_entry.get()
if file_path:
faces = detect_faces(file_path)
if len(faces) > 0:
# 在这里处理检测到的人脸并展示结果
pass
else:
self.label.config(text="No face detected.")
if __name__ == "__main__":
app = FaceRecognitionApp()
app.mainloop()
```
这个示例创建了一个简单GUI,用户可以选择一个图片文件,然后点击按钮执行人脸检测。实际的图像处理和人脸识别会更复杂,可能涉及到神经网络模型。
阅读全文
相关推荐
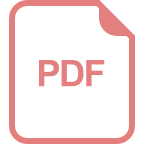
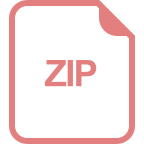
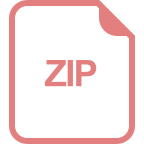
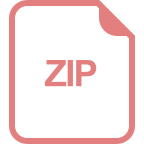
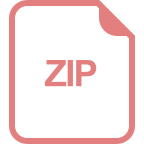
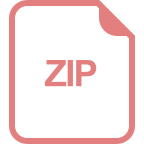
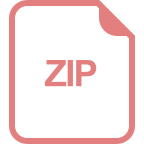
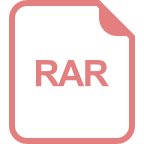
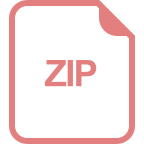
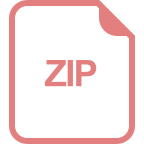
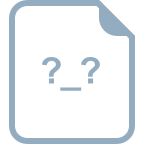
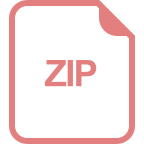
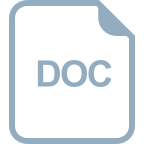
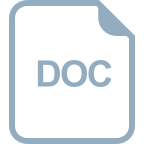
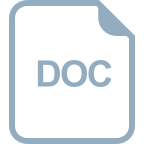
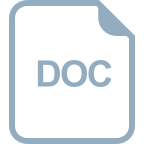
