java List的 stream 怎么用
时间: 2024-09-15 18:08:31 浏览: 34
在Java中,Stream API是一个强大的工具,用于处理集合(如List)中的数据。`List.stream()`方法会返回一个流(Stream),你可以对这个流执行一系列的中间操作(如map、filter、sorted等)和最终操作(如collect、forEach等),从而避免显式遍历整个列表。
例如,如果你有一个包含整数的List,你想计算所有偶数的和,可以这样做:
```java
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int sumOfEvens = numbers.stream()
.filter(n -> n % 2 == 0) // 过滤出偶数
.mapToInt(Integer::intValue) // 将Stream转换为IntStream便于求和
.sum(); // 计算和
```
在这个例子中,`filter`方法筛选出偶数,`mapToInt`将每个元素映射为它的整数值,然后`sum`方法计算总和。
相关问题
java liststream filter
Java中的List是一种常见的数据结构,用于存储一组元素。而List接口中的stream()方法可以将List转换为一个流(Stream),这样我们就可以使用Stream API对这个List进行一些处理。
在Stream API中,filter()方法是一个常用的操作,它可以根据指定条件过滤出符合条件的元素。例如,我们可以使用filter()方法从一个List中过滤出所有大于10的数字:
```
List<Integer> list = Arrays.asList(1, 5, 10, 15, 20);
List<Integer> filteredList = list.stream()
.filter(num -> num > 10)
.collect(Collectors.toList());
```
上述代码中,我们首先将一个数组转换为List,然后调用stream()方法将其转换为一个流。接着使用filter()方法过滤出大于10的数字,并使用collect()方法将过滤后的结果收集到一个新的List中。
java list stream treemap
Java List: A List is an interface in Java that extends the Collection interface. It is an ordered collection of elements that can have duplicates.
Java Stream: A Java Stream is a sequence of elements that can be processed in parallel or sequentially. Streams are used to manipulate collections of objects and can be used to perform operations on collections such as filtering, sorting, mapping, and more.
Java TreeMap: A TreeMap is a class in Java that implements the Map interface. It is a sorted map that stores key-value pairs in a tree structure. The keys are sorted in a natural order or by a specified Comparator. TreeMap is useful when you need to maintain a sorted order of keys.
Using Streams with List and TreeMap:
Java Stream can be used with List and TreeMap to perform various operations on the elements of these collections. Here are some examples:
1. Filtering elements from a List using Stream:
List<String> names = Arrays.asList("John", "Jane", "Mary", "Susan");
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("J"))
.collect(Collectors.toList());
In this example, we have a List of names and we are using a Stream to filter out the names that start with the letter "J".
2. Sorting elements in a TreeMap using Stream:
TreeMap<Integer, String> map = new TreeMap<>();
map.put(1, "John");
map.put(2, "Jane");
map.put(3, "Mary");
map.put(4, "Susan");
Map<Integer, String> sortedMap = map.entrySet().stream()
.sorted(Map.Entry.comparingByKey())
.collect(Collectors.toMap(Map.Entry::getKey, Map.Entry::getValue,
(oldValue, newValue) -> oldValue, LinkedHashMap::new));
In this example, we have a TreeMap with integer keys and string values. We are using a Stream to sort the key-value pairs by the key in ascending order and then store them in a new LinkedHashMap.
阅读全文
相关推荐
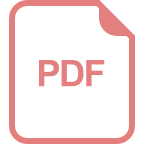
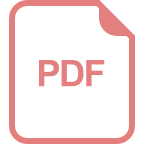
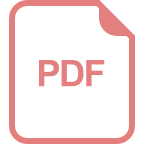













