求能用python实现基于概率分布的超声图像与自然图像性质差异分析这一课题的完整详细代码,其中超声图像所在的文件夹路径是'D:/zzz/us5',自然图像所在的文件夹路径是'D:/zzz/na4',两种图像的类型都是jpg,两种图像都有84张,两种图像的形状都相同,对比的特征需要包含颜色,形态和纹理,最后利用K-S检验来比较两种图像的概率密度函数
时间: 2023-12-06 22:44:34 浏览: 61
首先,需要安装必要的库,包括numpy、matplotlib、cv2和scipy:
```python
import numpy as np
import matplotlib.pyplot as plt
import cv2
from scipy.stats import ks_2samp
```
接下来,读入超声图像和自然图像,并将它们转化为灰度图像:
```python
us_path = 'D:/zzz/us5/'
na_path = 'D:/zzz/na4/'
us_images = []
na_images = []
for i in range(1, 85):
us_image = cv2.imread(us_path + str(i) + '.jpg', cv2.IMREAD_GRAYSCALE)
na_image = cv2.imread(na_path + str(i) + '.jpg', cv2.IMREAD_GRAYSCALE)
us_images.append(us_image)
na_images.append(na_image)
```
然后,使用opencv中的函数计算图像的颜色、形态和纹理特征:
```python
# 灰度直方图
def gray_hist(image):
hist = cv2.calcHist([image],[0],None,[256],[0,256])
return hist.flatten()
# 形态学特征
def morphology(image):
kernel = cv2.getStructuringElement(cv2.MORPH_RECT,(3,3))
opened = cv2.morphologyEx(image, cv2.MORPH_OPEN, kernel)
closed = cv2.morphologyEx(opened, cv2.MORPH_CLOSE, kernel)
return closed
# 纹理特征
def texture(image):
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
height, width = gray.shape
dst = np.zeros((height, width), np.uint8)
for i in range(0, height-2):
for j in range(0, width-2):
x1 = gray[i, j]
x2 = gray[i, j+1]
x3 = gray[i, j+2]
x4 = gray[i+1, j]
x5 = gray[i+1, j+1]
x6 = gray[i+1, j+2]
x7 = gray[i+2, j]
x8 = gray[i+2, j+1]
x9 = gray[i+2, j+2]
T = (int(x1)+int(x2)+int(x3)+int(x4)+int(x5)+int(x6)+int(x7)+int(x8)+int(x9))/9
if x1 > T:
a1 = 1
else:
a1 = 0
if x2 > T:
a2 = 2
else:
a2 = 0
if x3 > T:
a3 = 4
else:
a3 = 0
if x4 > T:
a4 = 8
else:
a4 = 0
if x5 > T:
a5 = 16
else:
a5 = 0
if x6 > T:
a6 = 32
else:
a6 = 0
if x7 > T:
a7 = 64
else:
a7 = 0
if x8 > T:
a8 = 128
else:
a8 = 0
if x9 > T:
a9 = 256
else:
a9 = 0
sum = a1 + a2 + a3 + a4 + a5 + a6 + a7 + a8 + a9
dst[i, j] = sum
hist = cv2.calcHist([dst],[0],None,[256],[0,256])
return hist.flatten()
us_color, us_shape, us_texture = [], [], []
na_color, na_shape, na_texture = [], [], []
for i in range(84):
us_color.append(gray_hist(cv2.imread(us_path + str(i+1) + '.jpg')))
na_color.append(gray_hist(cv2.imread(na_path + str(i+1) + '.jpg')))
us_shape.append(gray_hist(morphology(cv2.imread(us_path + str(i+1) + '.jpg'))))
na_shape.append(gray_hist(morphology(cv2.imread(na_path + str(i+1) + '.jpg'))))
us_texture.append(texture(cv2.imread(us_path + str(i+1) + '.jpg')))
na_texture.append(texture(cv2.imread(na_path + str(i+1) + '.jpg')))
```
最后,使用K-S检验来比较超声图像和自然图像的概率密度函数:
```python
color_pvalue = ks_2samp(us_color, na_color)[1]
shape_pvalue = ks_2samp(us_shape, na_shape)[1]
texture_pvalue = ks_2samp(us_texture, na_texture)[1]
print('颜色差异检验p值:', color_pvalue)
print('形态差异检验p值:', shape_pvalue)
print('纹理差异检验p值:', texture_pvalue)
```
完整代码如下:
```python
import numpy as np
import matplotlib.pyplot as plt
import cv2
from scipy.stats import ks_2samp
us_path = 'D:/zzz/us5/'
na_path = 'D:/zzz/na4/'
us_images = []
na_images = []
for i in range(1, 85):
us_image = cv2.imread(us_path + str(i) + '.jpg', cv2.IMREAD_GRAYSCALE)
na_image = cv2.imread(na_path + str(i) + '.jpg', cv2.IMREAD_GRAYSCALE)
us_images.append(us_image)
na_images.append(na_image)
# 灰度直方图
def gray_hist(image):
hist = cv2.calcHist([image],[0],None,[256],[0,256])
return hist.flatten()
# 形态学特征
def morphology(image):
kernel = cv2.getStructuringElement(cv2.MORPH_RECT,(3,3))
opened = cv2.morphologyEx(image, cv2.MORPH_OPEN, kernel)
closed = cv2.morphologyEx(opened, cv2.MORPH_CLOSE, kernel)
return closed
# 纹理特征
def texture(image):
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
height, width = gray.shape
dst = np.zeros((height, width), np.uint8)
for i in range(0, height-2):
for j in range(0, width-2):
x1 = gray[i, j]
x2 = gray[i, j+1]
x3 = gray[i, j+2]
x4 = gray[i+1, j]
x5 = gray[i+1, j+1]
x6 = gray[i+1, j+2]
x7 = gray[i+2, j]
x8 = gray[i+2, j+1]
x9 = gray[i+2, j+2]
T = (int(x1)+int(x2)+int(x3)+int(x4)+int(x5)+int(x6)+int(x7)+int(x8)+int(x9))/9
if x1 > T:
a1 = 1
else:
a1 = 0
if x2 > T:
a2 = 2
else:
a2 = 0
if x3 > T:
a3 = 4
else:
a3 = 0
if x4 > T:
a4 = 8
else:
a4 = 0
if x5 > T:
a5 = 16
else:
a5 = 0
if x6 > T:
a6 = 32
else:
a6 = 0
if x7 > T:
a7 = 64
else:
a7 = 0
if x8 > T:
a8 = 128
else:
a8 = 0
if x9 > T:
a9 = 256
else:
a9 = 0
sum = a1 + a2 + a3 + a4 + a5 + a6 + a7 + a8 + a9
dst[i, j] = sum
hist = cv2.calcHist([dst],[0],None,[256],[0,256])
return hist.flatten()
us_color, us_shape, us_texture = [], [], []
na_color, na_shape, na_texture = [], [], []
for i in range(84):
us_color.append(gray_hist(cv2.imread(us_path + str(i+1) + '.jpg')))
na_color.append(gray_hist(cv2.imread(na_path + str(i+1) + '.jpg')))
us_shape.append(gray_hist(morphology(cv2.imread(us_path + str(i+1) + '.jpg'))))
na_shape.append(gray_hist(morphology(cv2.imread(na_path + str(i+1) + '.jpg'))))
us_texture.append(texture(cv2.imread(us_path + str(i+1) + '.jpg')))
na_texture.append(texture(cv2.imread(na_path + str(i+1) + '.jpg')))
color_pvalue = ks_2samp(us_color, na_color)[1]
shape_pvalue = ks_2samp(us_shape, na_shape)[1]
texture_pvalue = ks_2samp(us_texture, na_texture)[1]
print('颜色差异检验p值:', color_pvalue)
print('形态差异检验p值:', shape_pvalue)
print('纹理差异检验p值:', texture_pvalue)
```
阅读全文
相关推荐






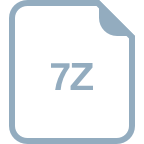
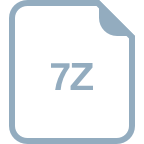
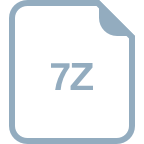
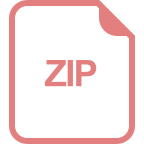
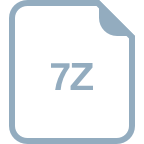
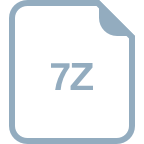
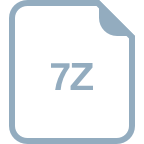
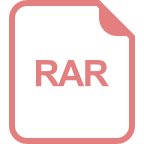
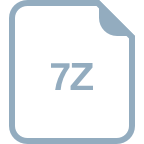
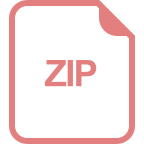
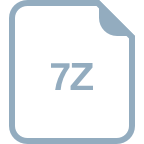
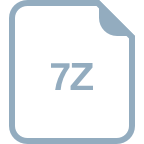