按以下提示信息,由基类的设计和测试开始,逐渐地完成各个类的设计,并且完成要求的功能。 ①设计一个Point(点)类,包含数据成员x、y(坐标点)。 ②以Point为基类,派生出一个Circle(圆)类,增加数据成员r(半径)。 ③以Circle类为直接基类,派生出一个Cylinder(圆柱体)类,再增加数据成员h(高)。 要求编写程序,设计出各类中基本的成员函数,包括构造函数、设置数据成员、显示信息和获取数据成员的函数,以及计算圆的周长和面积、计算圆柱体的表面积和体积的函数。
时间: 2024-02-11 09:03:55 浏览: 97
好的,根据您的要求,我可以为您提供以下代码示例:
```cpp
#include <iostream>
#include <cmath>
using namespace std;
const double PI = 3.1415926;
class Point {
protected:
double x, y;
public:
Point(double px, double py) : x(px), y(py) {}
void SetPoint(double px, double py) {
x = px;
y = py;
}
void ShowPoint() {
cout << "(" << x << ", " << y << ")";
}
double GetX() { return x; }
double GetY() { return y; }
};
class Circle : public Point {
protected:
double r;
public:
Circle(double px, double py, double pr) : Point(px, py), r(pr) {}
void SetCircle(double px, double py, double pr) {
SetPoint(px, py);
r = pr;
}
void ShowCircle() {
cout << "Center: ";
ShowPoint();
cout << ", Radius: " << r;
}
double GetRadius() { return r; }
double GetCircumference() { return 2 * PI * r; }
double GetArea() { return PI * r * r; }
};
class Cylinder : public Circle {
private:
double h;
public:
Cylinder(double px, double py, double pr, double ph) : Circle(px, py, pr), h(ph) {}
void SetCylinder(double px, double py, double pr, double ph) {
SetCircle(px, py, pr);
h = ph;
}
void ShowCylinder() {
cout << "Center: ";
ShowPoint();
cout << ", Radius: " << GetRadius() << ", Height: " << h;
}
double GetHeight() { return h; }
double GetSurfaceArea() { return GetCircumference() * h + 2 * GetArea(); }
double GetVolume() { return GetArea() * h; }
};
int main() {
Circle c(0, 0, 5);
Cylinder cy(0, 0, 5, 10);
c.ShowCircle();
cout << endl;
cy.ShowCylinder();
cout << endl;
cout << "Circle circumference: " << c.GetCircumference() << endl;
cout << "Circle area: " << c.GetArea() << endl;
cout << "Cylinder surface area: " << cy.GetSurfaceArea() << endl;
cout << "Cylinder volume: " << cy.GetVolume() << endl;
return 0;
}
```
以上代码定义了 Point、Circle 和 Cylinder 三个类,分别表示点、圆和圆柱体。其中 Point 类包含 x 和 y 两个数据成员,SetPoint() 函数用于设置坐标,ShowPoint() 函数用于显示坐标。Circle 类继承自 Point 类,增加了半径数据成员 r,并且重写了 SetPoint() 和 ShowPoint() 函数以适应圆的特点。Cylinder 类继承自 Circle 类,增加了高度数据成员 h,并且重写了 SetCircle() 和 ShowCircle() 函数以适应圆柱体的特点。此外,三个类分别实现了计算周长、面积、表面积和体积的函数。
在主函数中,我们实例化了一个圆和一个圆柱体,并分别调用它们的 Show() 函数以显示信息,同时计算并输出了它们的周长、面积、表面积和体积。
阅读全文
相关推荐
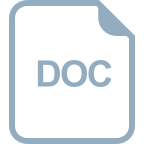
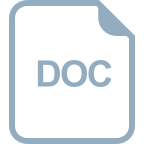
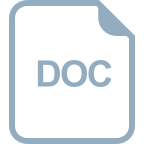

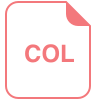
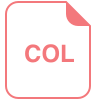
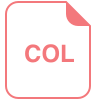
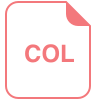
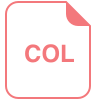
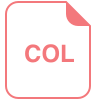
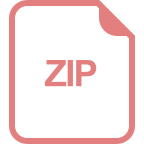
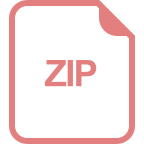
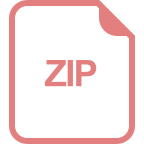
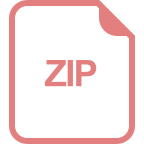
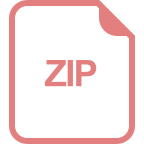
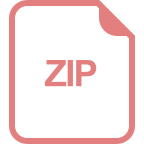