有一段英文由若干个单词组成,单词之间用空格分隔,编写程序提取其中所有的单词
时间: 2024-05-01 11:19:13 浏览: 11
假设该英文文本存储在字符串变量 `text` 中,可以使用正则表达式匹配所有单词,例如:
```python
import re
text = "Hello world! This is a test text."
words = re.findall(r'\b\w+\b', text)
print(words)
```
输出结果为:
```
['Hello', 'world', 'This', 'is', 'a', 'test', 'text']
```
其中正则表达式 `\b\w+\b` 匹配一个单词,`\b` 表示单词的边界,`\w+` 匹配一个或多个字母或数字。`re.findall` 方法返回所有匹配的结果。
相关问题
有一段英文有若干单词组成,单词之间用一个空格分隔,编写程序提取所有单词
假设该段英文存在字符串变量 `text` 中,可以使用以下 Python 代码提取所有单词:
```python
# 使用正则表达式匹配所有单词
import re
words = re.findall(r'\b\w+\b', text)
# 输出所有单词
for word in words:
print(word)
```
解释:
1. `re.findall()` 方法可以通过正则表达式匹配字符串中所有符合条件的子字符串,并以列表形式返回;
2. `\b` 表示单词的边界,`\w` 表示单词字符(包括字母、数字、下划线),`+` 表示匹配一个或多个;
3. 因此正则表达式 `\b\w+\b` 可以匹配所有单词;
4. 使用 `for` 循环遍历列表,输出每个单词。
有一段英文有若干单词组成,单词之间用一个空格分隔,用C语言编写程序提取所有单词
可以使用C语言中的字符串处理函数和循环来逐个字符读取输入字符串,并将单词逐个存储到一个数组中。以下是一个示例程序:
```c
#include <stdio.h>
#include <string.h>
#define MAX_WORDS 100
#define MAX_WORD_LEN 20
int main() {
char input_str[] = "Hello world! This is a test string.";
char words[MAX_WORDS][MAX_WORD_LEN];
int word_count = 0;
int word_len = 0;
int i;
// Loop through each character in the input string
for (i = 0; i < strlen(input_str); i++) {
char c = input_str[i];
// If the current character is a space or punctuation, then we have a complete word
if (c == ' ' || c == '\t' || c == '\n' || c == ',' || c == '.' || c == '?' || c == '!') {
if (word_len > 0) {
// Store the current word in the array
strncpy(words[word_count], &input_str[i - word_len], word_len);
words[word_count][word_len] = '\0';
word_count++;
word_len = 0;
}
} else {
// Add the current character to the current word
if (word_len < MAX_WORD_LEN - 1) {
words[word_count][word_len] = c;
word_len++;
}
}
// Stop processing if we have reached the maximum number of words
if (word_count >= MAX_WORDS) {
break;
}
}
// Print out all of the words
for (i = 0; i < word_count; i++) {
printf("%s\n", words[i]);
}
return 0;
}
```
在示例程序中,我们首先定义了一个输入字符串`input_str`和一个二维字符数组`words`用于存储所有单词。我们还定义了两个常量`MAX_WORDS`和`MAX_WORD_LEN`,分别表示最大单词数和每个单词的最大长度。
接下来,在循环中逐个字符读取输入字符串。如果当前字符是空格或标点符号,则表示当前单词已经结束,我们将其存储到数组中。否则,我们将当前字符添加到当前单词中。
最后,我们打印出所有的单词。注意,由于我们在存储每个单词时添加了一个字符串结束符`\0`,因此我们可以直接使用`printf`函数打印出每个单词。
相关推荐
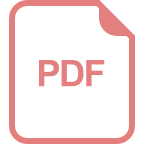
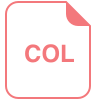
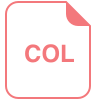
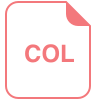
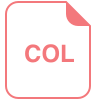
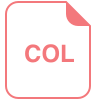







