具体代码
时间: 2023-07-03 16:22:18 浏览: 101
这里提供一个使用LSTM模型与GAN模型结合生成音乐和弦的示例代码,仅供参考:
```python
import numpy as np
import tensorflow as tf
from tensorflow.keras.layers import Input, LSTM, Dense
from tensorflow.keras.models import Model
from tensorflow.keras.optimizers import Adam
from tensorflow.keras.initializers import RandomNormal
# LSTM模型
def build_lstm_model(seq_length, n_notes, n_layers=1, hidden_size=128):
inputs = Input(shape=(seq_length, n_notes))
x = inputs
for i in range(n_layers):
x = LSTM(hidden_size, return_sequences=True)(x)
outputs = Dense(n_notes, activation='softmax')(x)
model = Model(inputs=inputs, outputs=outputs)
optimizer = Adam(lr=0.001)
model.compile(loss='categorical_crossentropy', optimizer=optimizer)
return model
# GAN模型
def build_gan_model(seq_length, n_notes, n_layers=1, hidden_size=128):
# 生成器
generator_inputs = Input(shape=(seq_length, n_notes))
x = generator_inputs
for i in range(n_layers):
x = LSTM(hidden_size, return_sequences=True)(x)
generator_outputs = Dense(n_notes, activation='softmax')(x)
generator = Model(inputs=generator_inputs, outputs=generator_outputs)
# 鉴别器
discriminator_inputs = Input(shape=(seq_length, n_notes))
x = discriminator_inputs
for i in range(n_layers):
x = LSTM(hidden_size, return_sequences=True)(x)
x = tf.keras.layers.GlobalAveragePooling1D()(x)
x = Dense(1, activation='sigmoid')(x)
discriminator = Model(inputs=discriminator_inputs, outputs=x)
discriminator.compile(loss='binary_crossentropy', optimizer=Adam(lr=0.0002, beta_1=0.5))
# GAN
discriminator.trainable = False
gan_inputs = Input(shape=(seq_length, n_notes))
gan_outputs = discriminator(generator(gan_inputs))
gan = Model(inputs=gan_inputs, outputs=gan_outputs)
gan.compile(loss='binary_crossentropy', optimizer=Adam(lr=0.0002, beta_1=0.5))
return generator, discriminator, gan
# 训练GAN模型
def train_gan(seq_length, n_notes, epochs=5000, batch_size=64):
# 加载训练数据
X_train = np.load('X_train.npy')
Y_train = np.load('Y_train.npy')
# 构建模型
generator, discriminator, gan = build_gan_model(seq_length, n_notes)
# 训练模型
for epoch in range(epochs):
# 训练鉴别器
idx = np.random.randint(0, X_train.shape[0], batch_size)
real_seqs = X_train[idx]
fake_seqs = generator.predict(np.random.randn(batch_size, seq_length, n_notes))
x = np.concatenate([real_seqs, fake_seqs])
y = np.zeros((2 * batch_size, 1))
y[:batch_size] = 1
y[batch_size:] = 0
d_loss = discriminator.train_on_batch(x, y)
# 训练生成器
x = np.random.randn(batch_size, seq_length, n_notes)
y = np.ones((batch_size, 1))
g_loss = gan.train_on_batch(x, y)
# 输出训练结果
if epoch % 100 == 0:
print('epoch: {}, d_loss: {}, g_loss: {}'.format(epoch, d_loss, g_loss))
# 保存模型
if epoch % 1000 == 0:
generator.save('generator_{}.h5'.format(epoch))
# 使用LSTM模型生成音乐
def generate_music_with_lstm(model, seed_notes, n_notes, seq_length, temperature=1.0):
generated_notes = []
input_notes = seed_notes
for i in range(n_notes):
# 预测下一个音符
x = np.reshape(input_notes, (1, seq_length, len(note_dict)))
y_pred = model.predict(x)[0]
# 根据温度调整预测结果,增加随机性
y_pred = np.log(y_pred) / temperature
y_pred = np.exp(y_pred) / np.sum(np.exp(y_pred))
# 选择下一个音符
idx = np.random.choice(len(note_dict), p=y_pred)
next_note = note_dict[idx]
generated_notes.append(next_note)
# 更新当前输入
input_notes = np.concatenate([input_notes[1:], np.eye(len(note_dict))[idx]])
return generated_notes
# 使用GAN模型生成音乐
def generate_music_with_gan(generator, seq_length, n_notes, temperature=1.0):
seed_noise = np.random.randn(1, seq_length, n_notes)
generated_notes = []
for i in range(n_notes):
# 生成下一个音符
x = generator.predict(seed_noise)[0]
# 根据温度调整预测结果,增加随机性
x = np.log(x) / temperature
x = np.exp(x) / np.sum(np.exp(x))
# 选择下一个音符
idx = np.random.choice(len(note_dict), p=x[-1])
next_note = note_dict[idx]
generated_notes.append(next_note)
# 更新当前输入
seed_noise = np.concatenate([seed_noise[:, 1:, :], np.eye(len(note_dict))[idx][np.newaxis, np.newaxis, :]], axis=1)
return generated_notes
```
以上代码中,`build_lstm_model()`函数用于构建LSTM模型,`build_gan_model()`函数用于构建GAN模型,`train_gan()`函数用于训练GAN模型,`generate_music_with_lstm()`函数用于使用LSTM模型生成音乐,`generate_music_with_gan()`函数用于使用GAN模型生成音乐。其中,`note_dict`为一个字典,将音符映射为一个整数,方便模型处理。
阅读全文
相关推荐
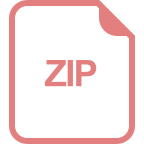
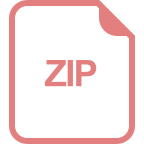
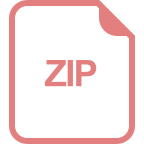
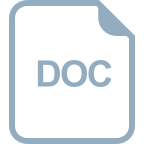
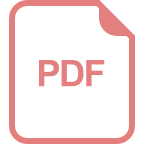
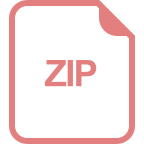
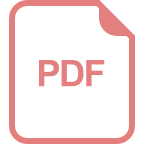
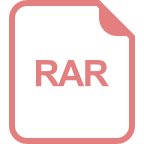