#include<reg51.h> void delay(int n) { int i,j; for(i = 0; i < n; i++) { for(j = 0; j < 20; j++) //循环20次,即为1s { while(TF0 == 0); TF0 = 0; TH0 = 0x3c; TL0 = 0xb0; } } } int a[8] = {1, 2, 4, 8, 16, 32, 64, 128}; void main() { int i = 0; TMOD = 0x01; //使用模式1 TH0 = 0x3c; //50ms TL0 = 0xb0; TR0 = 1; P2 = 1; while(1) //使P2八个引脚循环出现高电平 { P2 = a[i++]; delay(1); if(i == 8) { i = 0; } } }如何看/
时间: 2024-04-26 18:20:45 浏览: 18
理解这段代码?
这是一段使用 8051 单片机控制 P2 口输出高电平的代码。其中,delay 函数使用定时器模块实现了延时功能,每次延时 1 秒钟,而 main 函数中使用 while 循环将 P2 口的八个引脚循环输出高电平。具体实现方式是:定义一个数组 a 存储八个二进制数,然后使用一个循环将数组 a 中的数字依次输出到 P2 口。当输出完第八个数字后,将计数器 i 重置为 0,继续输出第一个数字,从而实现循环输出的功能。
相关问题
#include <reg51.h>代码
这是一段使用 Keil C51 编译器开发的 8051 单片机程序。`reg51.h` 是一个头文件,包含了 8051 单片机的寄存器定义及相关宏定义。具体代码实现需要根据具体需求进行编写。
以下是一个简单的例子,每隔一秒钟,P1 口输出高电平和低电平交替。
```c
#include <reg51.h>
void delay(unsigned int time) {
while(time--);
}
void main() {
while(1) {
P1 = 0xFF; // P1 输出高电平
delay(50000); // 延时 50000 次,约为 1 秒钟
P1 = 0x00; // P1 输出低电平
delay(50000); // 延时 50000 次,约为 1 秒钟
}
}
```
#include<REG52.H> #include<stdio.h> #include <intrins.h> sbit Trig=P2^0; sbit Echo=P2^1;超声模块测距代码补全
`#include<REG52.H>`是一段头文件的引用,代表使用了基于Intel 8051的单片机开发环境。`<stdio.h>`是标准输入输出头文件的引用。`<intrins.h>`是用于特殊函数的头文件引用。
以下是超声模块测距的代码补全:
```
#include<REG52.H>
#include<stdio.h>
#include <intrins.h>
sbit Trig=P2^0;
sbit Echo=P2^1;
void delay(unsigned int us) //延时函数
{
while(us--);
}
unsigned char read_distance() //读取距离函数
{
unsigned char distance;
Trig = 0; //初始化
delay(2);
Trig = 1; //触发测量
delay(10);
Trig = 0; //停止测量
while(!Echo); //等待Echo为高电平
TH0 = 0;
TL0 = 0; //清零计时器
while(Echo); //等待Echo为低电平
distance = (unsigned char)(TH0*256+TL0)/58.0; //计算距离
return distance;
}
void main()
{
unsigned char distance;
TMOD |= 0x01; //设置为定时器0,工作方式1
while(1)
{
distance = read_distance(); //读取距离
printf("The distance is %d cm\n",distance); //打印距离
delay(500); //延时500ms
}
}
```
相关推荐
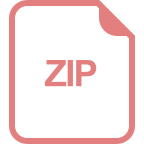
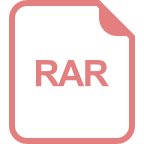













