帮我分析这段代码import xml.dom.minidom # 解析XML文件 doc = xml.dom.minidom.parse('test_Exp.xml') root = doc.documentElement # 选择要排序的字段 items = root.getElementsByTagName('Group') # 根据特定属性进行排序 items = sorted(items, key=lambda item: item.getAttribute('index')) new_root = doc.createElement('root') print(items) index = 1 for item in items: print(item.getAttribute('index')) item.setAttribute("index", index) print(item.getAttribute('index')) new_root.appendChild(item) index += 1 # 将新的XML节点添加到文档中 doc.removeChild(root) doc.appendChild(new_root) # 保存文档 with open('soted.xml', 'w', encoding='utf-8') as f: f.write(doc.toprettyxml(indent='\t', encoding='utf-8').decode('utf-8'))
时间: 2023-12-29 09:04:26 浏览: 33
这段代码是用来对一个 XML 文件中的特定元素进行排序,并将排序后的结果写入到新的 XML 文件中。具体实现步骤如下:
1. 使用 `xml.dom.minidom` 库的 `parse` 方法解析 XML 文件,得到文档对象 `doc`。
2. 通过 `doc.documentElement` 选择 XML 文件的根元素。在这个例子中,我们将对 `Group` 元素进行排序。
3. 使用 `root.getElementsByTagName('Group')` 方法选择所有的 `Group` 元素,并根据 `index` 属性进行排序。排序使用了 `sorted` 方法和 `key` 参数。
4. 创建一个新的根节点 `new_root`,并将排序后的 `Group` 元素依次添加到这个节点中。在添加元素时,我们使用 `setAttribute` 方法更新元素的 `index` 属性,并将元素添加到 `new_root` 中。
5. 使用 `doc.removeChild(root)` 方法删除原始的根节点。
6. 使用 `doc.appendChild(new_root)` 方法将新的根节点添加到文档对象中。
7. 最后,使用 `open` 函数打开一个新的文件,并使用 `doc.toprettyxml` 方法将文档对象转换为格式化的 XML 字符串,然后将这个字符串写入到文件中。
需要注意的是,在写入 XML 文件时,为了避免特殊字符导致的错误,代码对写入的内容进行了编码和解码操作。
相关问题
怎么使用xml.dom.minidom解析器来检查并修复报文的格式,确保它符合XML规范要求
你可以使用以下代码来解析和修复XML格式:
```
import xml.dom.minidom
# 读取XML文件
xml_file = open('example.xml', 'r')
xml_data = xml_file.read()
xml_file.close()
# 解析XML
try:
# 创建DOM文档对象
dom = xml.dom.minidom.parseString(xml_data)
# 检查XML格式
xml_data = dom.toprettyxml()
except Exception as e:
# 修复XML格式
xml_data = xml.dom.minidom.parseString('<root>' + xml_data + '</root>').toprettyxml()
# 保存XML文件
with open('example.xml', 'w') as xml_file:
xml_file.write(xml_data)
```
这段代码首先读取XML文件,然后使用`xml.dom.minidom`解析器来检查XML格式。如果XML格式正确,则直接通过`toprettyxml()`方法让XML文件格式更加美观。如果XML格式不正确,则使用`parseString()`方法将XML数据包装在一个`<root>`元素中,然后再使用`toprettyxml()`方法修复XML格式。最后,将修复后的XML文件保存回原文件。
如何安装xml.dom.minidom
1. 打开命令行窗口(Windows:按下Win+R组合键,输入cmd,按下回车;Mac:打开终端)。
2. 输入以下命令安装pip工具:
```
curl https://bootstrap.pypa.io/get-pip.py -o get-pip.py
python get-pip.py
```
3. 安装完成后,输入以下命令安装xml.dom.minidom:
```
pip install xml.dom.minidom
```
4. 安装完成后,可以在Python脚本中使用xml.dom.minidom模块。例如:
```
import xml.dom.minidom
# 创建一个空的文档对象
doc = xml.dom.minidom.Document()
# 创建根元素
root = doc.createElement('root')
doc.appendChild(root)
# 创建子元素
child1 = doc.createElement('child1')
root.appendChild(child1)
# 保存为xml文件
with open('output.xml', 'w') as f:
f.write(doc.toprettyxml())
```
这个例子创建了一个空的XML文档对象,并添加了一个根元素和一个子元素,最后将文档保存为output.xml文件。
相关推荐
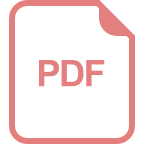
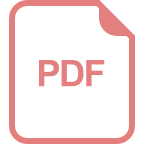












