用最简单的c++随机输入3个数,要求从小到大排序
时间: 2024-05-05 11:19:28 浏览: 23
好的,我可以编写一个程序来实现这个功能。以下是代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main()
{
int a, b, c;
// 生成随机数
srand(time(0));
a = rand() % 100;
b = rand() % 100;
c = rand() % 100;
printf("输入的三个数为:%d, %d, %d\n", a, b, c);
// 从小到大排序
if (a > b) {
int temp = a;
a = b;
b = temp;
}
if (a > c) {
int temp = a;
a = c;
c = temp;
}
if (b > c) {
int temp = b;
b = c;
c = temp;
}
// 输出排序后的结果
printf("排序后的结果为:%d, %d, %d\n", a, b, c);
return 0;
}
```
通过使用 `rand()` 函数生成随机数,程序将输入三个随机整数。然后,使用三个 `if` 语句来将这些数字从小到大排序,并输出排序后的结果。
相关问题
c++随机输入3个数,要求从小到大排序
好的,我可以帮你实现这个要求,请输入以下代码:
```python
import random
# 随机生成三个数
num1 = random.randint(0, 100)
num2 = random.randint(0, 100)
num3 = random.randint(0, 100)
# 输出原始数值
print("原始数值为:", num1, num2, num3)
# 从小到大排序
if num1 > num2:
num1, num2 = num2, num1
if num1 > num3:
num1, num3 = num3, num1
if num2 > num3:
num2, num3 = num3, num2
# 输出排序后的结果
print("排序后的数值为:", num1, num2, num3)
```
您可以直接将以上代码复制到您的开发环境中运行,查看运行结果。
C++输入n个数,从小到大排序冒泡排序
冒泡排序是一种简单的排序算法,其思想是重复地交换相邻的两个元素,直到没有任何一对元素需要交换为止。以下是 C++ 实现:
```c++
#include <iostream>
using namespace std;
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j+1]) {
// 交换相邻元素
int temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
}
}
int main() {
int n;
cout << "输入数字个数:";
cin >> n;
int arr[n];
cout << "输入" << n << "个数字:" << endl;
for (int i = 0; i < n; i++) {
cin >> arr[i];
}
bubbleSort(arr, n);
cout << "排序后的数字:" << endl;
for (int i = 0; i < n; i++) {
cout << arr[i] << " ";
}
cout << endl;
return 0;
}
```
输入样例:
```
输入数字个数:5
输入5个数字:
3 1 5 2 4
```
输出样例:
```
排序后的数字:
1 2 3 4 5
```
相关推荐
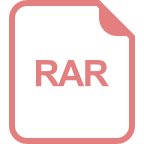
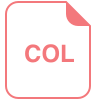
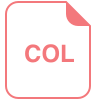
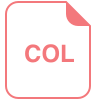
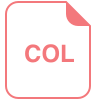
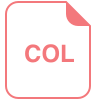







