7-12 jmu-Java\\&Python-统计文字中的单词数量并按出现次数排序
时间: 2024-05-16 13:16:12 浏览: 233
可以使用 Python 中的字典(dict)来实现单词数量的统计和排序。
首先,我们需要读入文本文件,并将其转换为一个字符串。可以使用 Python 中的 open 函数打开文件,并使用 read 方法读取文件内容。为了方便处理,我们可以将所有字母都转换为小写字母。
```python
with open('text.txt', 'r') as f:
text = f.read().lower()
```
接下来,我们需要将字符串拆分成单个单词,并统计它们的出现次数。可以使用 Python 中的 split 方法来拆分字符串,并使用一个字典来存储每个单词出现的次数。如果字典中已经存在该单词,则将其出现次数加一;否则,在字典中添加该单词并将其出现次数设置为一。
```python
word_count = {}
for word in text.split():
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
```
最后,我们可以根据单词出现的次数对字典进行排序,并将结果打印出来。可以使用 Python 中的 sorted 函数和 lambda 表达式来实现排序。
```python
sorted_word_count = sorted(word_count.items(), key=lambda x: x[1], reverse=True)
for word, count in sorted_word_count:
print(f'{word}: {count}')
```
完整代码如下:
```python
with open('text.txt', 'r') as f:
text = f.read().lower()
word_count = {}
for word in text.split():
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
sorted_word_count = sorted(word_count.items(), key=lambda x: x[1], reverse=True)
for word, count in sorted_word_count:
print(f'{word}: {count}')
```
其中,text.txt 是要统计的文本文件的文件名。
阅读全文
相关推荐






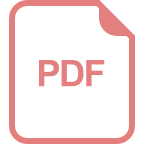
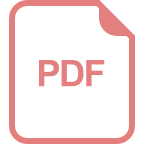
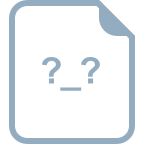
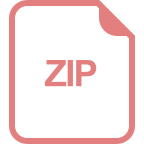
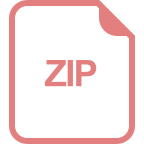
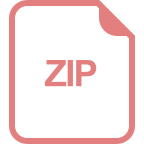
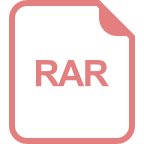
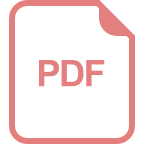