用jupyter写一个简单的图片识别界面,模型类型为pth
时间: 2023-06-13 12:07:16 浏览: 109
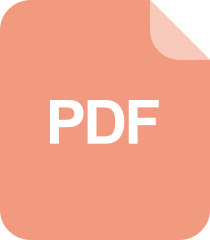
Python编程实现的图片识别功能示例
首先,需要安装必要的库,包括PyTorch、numpy、Pillow以及ipywidgets。可以使用以下代码进行安装:
```
!pip install torch numpy Pillow ipywidgets
```
然后,需要加载训练好的模型,可以使用以下代码:
```
import torch
# 加载模型
model = torch.load('model.pth')
```
接下来,需要编写一个函数来对输入的图片进行预测并显示结果。代码如下:
```
from PIL import Image
import numpy as np
import ipywidgets as widgets
from IPython.display import display
# 定义分类名称
classes = ['cat', 'dog']
# 定义预测函数
def predict(image_path):
# 打开图片并进行预处理
image = Image.open(image_path)
image = image.resize((224, 224))
image = np.array(image) / 255.0
image = (image - np.array([0.485, 0.456, 0.406])) / np.array([0.229, 0.224, 0.225])
image = image.transpose((2, 0, 1))
image = np.expand_dims(image, axis=0)
image = torch.tensor(image, dtype=torch.float32)
# 进行预测
with torch.no_grad():
output = model(image)
output = torch.softmax(output, dim=1)
class_index = output.argmax()
# 显示结果
result = classes[class_index]
print('Prediction:', result)
# 显示图片
display(Image.open(image_path))
```
最后,可以使用ipywidgets库创建一个文件选择器和按钮,让用户可以选择图片并进行预测。代码如下:
```
file_selector = widgets.FileUpload()
predict_button = widgets.Button(description='Predict')
def on_predict_button_clicked(button):
# 获取上传的文件并进行预测
for file_name in file_selector.value.keys():
with open(file_name, 'wb') as f:
f.write(file_selector.value[file_name]['content'])
predict(file_name)
predict_button.on_click(on_predict_button_clicked)
display(file_selector)
display(predict_button)
```
完成以上步骤后,就可以在Jupyter Notebook中创建一个简单的图片识别界面了。用户可以选择一张图片,点击预测按钮,模型会对图片进行预测并显示结果。需要注意的是,此代码仅适用于单张图片的预测,如果需要对多张图片进行预测,需要对代码进行相应的修改。
阅读全文
相关推荐
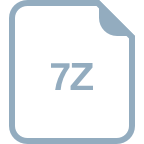


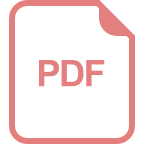
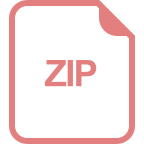
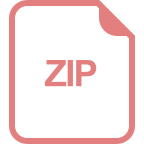
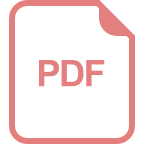
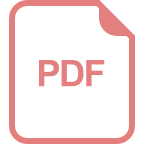
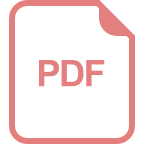