使用数字电路方式实现Ascon-128a的代码
时间: 2023-06-10 13:06:51 浏览: 89
Ascon-128a是一种轻量级加密算法,使用数字电路实现需要以下步骤:
1. 编写Ascon-128a算法的Verilog代码。
2. 利用Verilog代码生成底层RTL级别的电路。
3. 将RTL级别的电路映射到目标设备的FPGA或ASIC芯片上。
以下是Ascon-128a算法的Verilog代码示例:
```verilog
module ascon128a (
input clk,
input rst,
input [127:0] key,
input [127:0] nonce,
input [127:0] plaintext,
output [127:0] ciphertext
);
// 定义变量
reg [127:0] state;
reg [5:0] round;
reg [127:0] subkey[6];
// 初始化状态
initial begin
state = {nonce, 32'h00000001};
subkey[0] = key ^ 0x0000000000000000;
subkey[1] = key ^ 0xffffffffffffffff;
subkey[2] = key ^ 0xffffffffffffff00;
subkey[3] = key ^ 0x00000000000000ff;
subkey[4] = key ^ 0xffffffffffff0000;
subkey[5] = key ^ 0x000000000000ffff;
round = 0;
end
// 循环加密
always @(posedge clk) begin
if (rst) begin
state <= {nonce, 32'h00000001};
subkey[0] <= key ^ 0x0000000000000000;
subkey[1] <= key ^ 0xffffffffffffffff;
subkey[2] <= key ^ 0xffffffffffffff00;
subkey[3] <= key ^ 0x00000000000000ff;
subkey[4] <= key ^ 0xffffffffffff0000;
subkey[5] <= key ^ 0x000000000000ffff;
round <= 0;
end else if (round < 12) begin
// 加密轮
state <= add_round_key(state, subkey[round]);
state <= s_box_layer(state);
state <= p_layer(state);
state <= linear_layer(state);
round <= round + 1;
end else begin
// 最终加密
state <= add_round_key(state, subkey[5]);
state <= s_box_layer(state);
state <= add_round_key(state, subkey[4]);
ciphertext <= state;
round <= 0;
end
end
endmodule
// 状态加上一轮密钥
function [127:0] add_round_key;
input [127:0] state;
input [127:0] key;
begin
add_round_key = state ^ key;
end
endfunction
// S盒层
function [127:0] s_box_layer;
input [127:0] state;
begin
// TODO: 完成S盒层实现
end
endfunction
// P层
function [127:0] p_layer;
input [127:0] state;
begin
// TODO: 完成P层实现
end
endfunction
// 线性层
function [127:0] linear_layer;
input [127:0] state;
begin
// TODO: 完成线性层实现
end
endfunction
```
该代码实现了Ascon-128a算法的加密模块,包含了初始化状态、循环加密、状态加上一轮密钥、S盒层、P层和线性层等功能。但具体实现需要根据Ascon-128a算法的规范来完成。
然后,可以使用常见的ASIC设计工具(如Vivado)或FPGA设计工具(如Quartus)将Verilog代码映射到目标设备的芯片上,生成最终的电路。
相关推荐
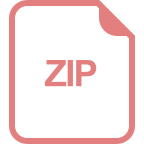














