C++/CLI 如何封装VB.NET中使用的带结构体的Windows 的API,在VB.NET中调用封装后的Api,详细例子
时间: 2024-03-27 21:36:22 浏览: 27
首先,需要编写 C++/CLI 封装带结构体的 Windows API,然后在 VB.NET 中调用该封装后的 API。
以下是一个简单的例子,演示如何封装 `CreateFile` 函数:
C++/CLI 封装:
```cpp
#pragma once
#include <Windows.h>
using namespace System;
using namespace System::Runtime::InteropServices;
namespace MyAPIWrapper
{
public ref class FileHandleWrapper
{
public:
property IntPtr Handle
{
IntPtr get() { return m_handle; }
}
FileHandleWrapper(String^ fileName, FileMode mode, FileAccess access)
{
pin_ptr<const wchar_t> pFileName = PtrToStringChars(fileName);
DWORD dwDesiredAccess = 0;
DWORD dwCreationDisposition = 0;
switch (access)
{
case FileAccess::Read:
dwDesiredAccess = GENERIC_READ;
break;
case FileAccess::Write:
dwDesiredAccess = GENERIC_WRITE;
break;
case FileAccess::ReadWrite:
dwDesiredAccess = GENERIC_READ | GENERIC_WRITE;
break;
}
switch (mode)
{
case FileMode::Create:
dwCreationDisposition = CREATE_ALWAYS;
break;
case FileMode::Open:
dwCreationDisposition = OPEN_EXISTING;
break;
}
m_handle = CreateFile(pFileName, dwDesiredAccess, 0, NULL, dwCreationDisposition, 0, NULL);
}
~FileHandleWrapper()
{
CloseHandle(m_handle);
}
private:
IntPtr m_handle;
};
}
```
VB.NET 调用:
```vb
Imports System.IO
Imports MyAPIWrapper
Public Class Form1
Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click
Dim fileName As String = "test.txt"
Dim mode As FileMode = FileMode.Create
Dim access As FileAccess = FileAccess.Write
Using fh As New FileHandleWrapper(fileName, mode, access)
Using sw As New StreamWriter(New FileStream(fh.Handle, FileAccess.Write))
sw.WriteLine("Hello, world!")
End Using
End Using
End Sub
End Class
```
在 VB.NET 中,我们可以像使用普通的文件句柄一样使用封装后的 `FileHandleWrapper` 类,这样可以避免直接调用 Windows API 带来的一些问题,同时也方便了 VB.NET 开发人员的使用。
相关推荐
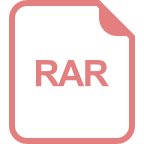
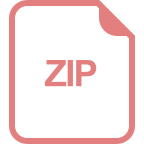














