document.addEventListener('DOMContentLoaded', function() { // 获取按钮和输入框的元素 const generateBtn = document.getElementById('10'); const phoneInput = document.getElementById('7'); const verifyInput = document.getElementById('8'); const submitBtn = document.getElementById('submit-btn'); let randomNum = null; // 存储随机数的变量 // 生成随机数并弹窗显示 generateBtn.addEventListener('click', function(event) { event.preventDefault(); // 如果手机号不满足要求,则弹出手机号错误的提示框 if (!validatePhone()) { alert('手机号错误'); return; } // 如果随机数还未生成,则生成新的随机数 if (randomNum === null) { randomNum = Math.floor(Math.random() * 10000); } // 弹窗显示随机数 alert('验证码:' + randomNum); }); // 提交按钮点击事件监听器 submitBtn.addEventListener('click', function(event) { // 获取存储的随机数 const storedRandomNum = randomNum; // 获取用户输入的验证码和手机号 const userEnteredNum = parseInt(verifyInput.value); const phoneValue = phoneInput.value; // 检查是否已生成随机数 if (storedRandomNum === null) { event.preventDefault(); } else if (userEnteredNum !== storedRandomNum) { // 检查验证码是否正确 event.preventDefault(); alert('验证码错误'); } else { // 验证码正确,提交表单 // 这里可以添加其他逻辑或调用其他函数来处理表单提交 } }); // 手机号验证函数 function validatePhone() { const phoneValue = phoneInput.value; // 检查手机号是否为11个数字 return /^\d{11}$/.test(phoneValue); } }); 为什么刚进入界面时会弹出”手机号错误“的弹窗
时间: 2024-04-15 09:24:51 浏览: 146
刚进入界面时会弹出"手机号错误"的弹窗,是因为在点击生成随机数按钮之前,会先调用手机号验证函数 validatePhone() 来检查手机号是否满足要求。
如果手机号不满足要求,即不是11个数字,那么就会触发 alert('手机号错误'),弹出"手机号错误"的提示框。
这样做是为了确保用户输入的手机号是有效的,只有在手机号满足要求时才能生成随机数。
阅读全文
相关推荐
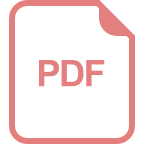
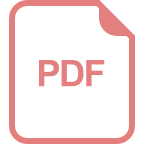
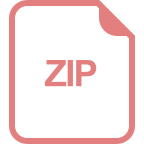
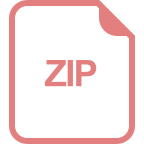
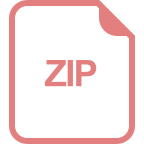
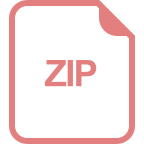
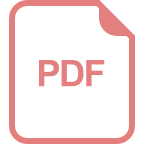
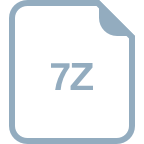
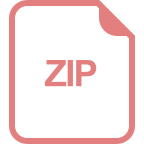
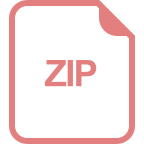
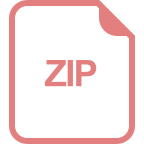
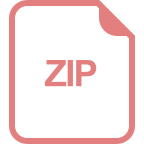
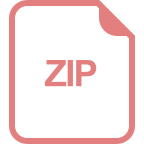
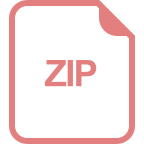
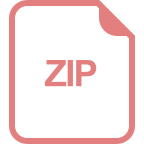
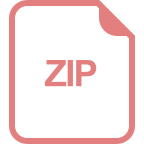
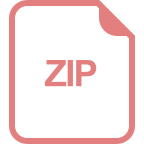