写一个纯前端的模糊查询农产品的功能,需要在查询框里没有内容的时候,显示全部商品
时间: 2024-05-01 12:22:50 浏览: 46
好的,这里提供一种基于Vue.js的实现方式。
首先,需要准备一份农产品数据,可以是一个JSON文件,如下所示:
```json
[
{ "name": "苹果", "price": 5.5 },
{ "name": "香蕉", "price": 3.2 },
{ "name": "橙子", "price": 4.0 },
{ "name": "西瓜", "price": 10.0 }
]
```
然后,在Vue组件中,定义一个data属性存储查询框中的关键词和商品列表:
```html
<template>
<div>
<input type="text" v-model="keyword" @input="handleInput" />
<ul>
<li v-for="product in products" :key="product.name">
{{ product.name }} - {{ product.price }}
</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
keyword: '',
products: []
}
},
methods: {
handleInput() {
// 获取关键词
const keyword = this.keyword.trim().toLowerCase()
if (keyword === '') {
// 如果关键词为空,则显示全部商品
this.products = this.allProducts
} else {
// 根据关键词过滤商品
this.products = this.allProducts.filter((product) =>
product.name.toLowerCase().includes(keyword)
)
}
}
},
mounted() {
// 加载全部商品数据
this.allProducts = [
{ name: '苹果', price: 5.5 },
{ name: '香蕉', price: 3.2 },
{ name: '橙子', price: 4.0 },
{ name: '西瓜', price: 10.0 }
]
this.products = this.allProducts
}
}
</script>
```
上面的代码中,handleInput方法在关键词发生变化时被调用,根据关键词过滤商品并更新列表。如果关键词为空,则显示全部商品。
mounted方法在组件加载时被调用,加载全部商品数据并初始化列表。
最后,将组件添加到页面中即可:
```html
<div id="app">
<product-list></product-list>
</div>
<script>
import ProductList from './ProductList.vue'
new Vue({
el: '#app',
components: {
ProductList
}
})
</script>
```
阅读全文
相关推荐
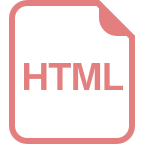
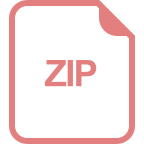
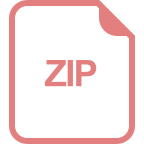















