用c语言编写,从键盘输入一个字符串,统计按a到z各个字母的出现字数
时间: 2024-05-03 21:21:06 浏览: 100
OK,请输入代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[100]; // 假设字符串长度不超过100
int len, count[26] = {0}; // count数组存放按a到z的字母出现次数
printf("请输入一个字符串:\n");
fgets(str, sizeof(str), stdin); // 从键盘读入字符串
len = strlen(str); // 获取字符串长度
for (int i = 0; i < len; i++) {
if (str[i] >= 'a' && str[i] <= 'z') { // 判断字符是否为小写字母
count[str[i] - 'a']++; // 字符出现次数加一
}
}
// 输出a到z各字母出现次数
for (int i = 0; i < 26; i++) {
printf("%c:%d\n", 'a' + i, count[i]);
}
return 0;
}
```
如果你想使用更好的观感,可以在个别系统上使用:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[100]; // 假设字符串长度不超过100
int len, count[26] = {0}; // count数组存放按a到z的字母出现次数
printf("请输入一个字符串:\n");
fgets(str, sizeof(str), stdin); // 从键盘读入字符串
len = strlen(str); // 获取字符串长度
for (int i = 0; i < len; i++) {
if (str[i] >= 'a' && str[i] <= 'z') { // 判断字符是否为小写字母
count[str[i] - 'a']++; // 字符出现次数加一
}
}
// 打印表头
printf("+-----+-----+\n");
printf("|%-5s|%-5s|\n", "字母", "出现次数");
printf("+-----+-----+\n");
// 输出a到z各字母出现次数
for (int i = 0; i < 26; i++) {
printf("|%-5c|%-5d|\n", 'a' + i, count[i]);
printf("+-----+-----+\n");
}
return 0;
}
```
阅读全文
相关推荐
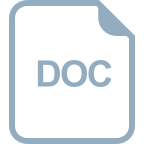
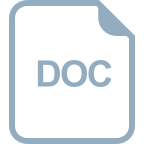
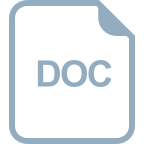
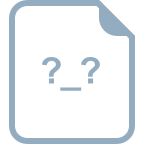
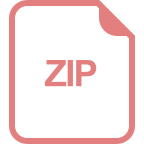
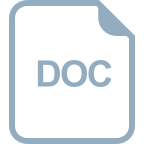
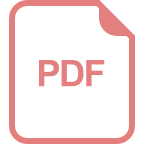
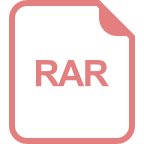
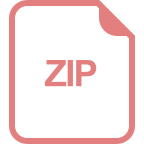
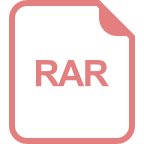
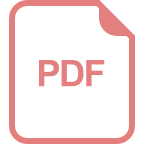
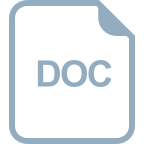