C++手动输入一个由0和1组成的数组,计算全为1的最大矩形的面积
时间: 2023-11-22 16:55:25 浏览: 71
这是一个经典的算法问题,可以用栈来解决。以下是C++代码实现:
```c++
#include <iostream>
#include <stack>
using namespace std;
int getMaxArea(int hist[], int n) {
stack<int> s;
int max_area = 0;
int tp;
int area_with_top;
int i = 0;
while (i < n) {
if (s.empty() || hist[s.top()] <= hist[i])
s.push(i++);
else {
tp = s.top();
s.pop();
area_with_top = hist[tp] * (s.empty() ? i : i - s.top() - 1);
if (max_area < area_with_top)
max_area = area_with_top;
}
}
while (!s.empty()) {
tp = s.top();
s.pop();
area_with_top = hist[tp] * (s.empty() ? i : i - s.top() - 1);
if (max_area < area_with_top)
max_area = area_with_top;
}
return max_area;
}
int main() {
int n;
cout << "请输入数组长度:";
cin >> n;
int hist[n];
cout << "请输入由0和1组成的数组:" << endl;
for (int i = 0; i < n; i++) {
cin >> hist[i];
}
cout << "全为1的最大矩形的面积为:" << getMaxArea(hist, n) << endl;
return 0;
}
```
在这个代码中,我们使用了一个栈来存储每个矩形的高度。我们遍历整个数组,如果当前的高度大于等于栈顶元素,则将当前索引压入栈中;否则,我们弹出栈顶元素,并计算以该元素为高度的矩形的面积。在计算矩形面积时,我们需要注意矩形的宽度是由左侧第一个高度小于该元素的索引和右侧第一个高度小于该元素的索引决定的。最后,我们将最大面积返回。
注意,这个算法的时间复杂度为O(n),其中n为数组长度。
阅读全文
相关推荐
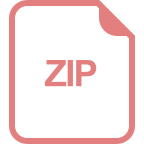
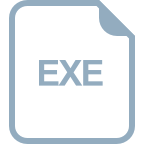
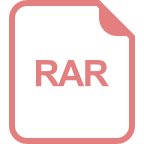
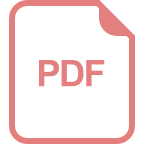
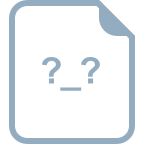
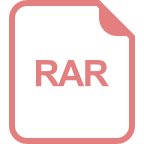
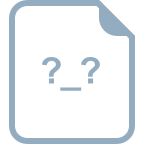
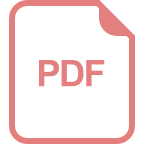
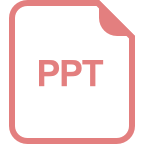
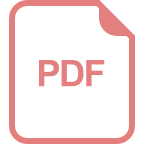
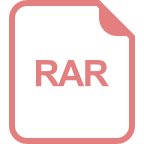
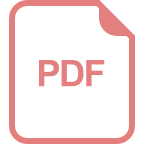