andserver websocket
时间: 2023-05-03 09:03:51 浏览: 136
AndServer WebSocket是一种基于HTTP协议的协议,它允许在客户端和服务器之间进行实时的双向数据通信。AndServer是一款开源的Android Web服务器,它支持HTTP和HTTPS协议,同时也提供了WebSocket的支持。
和传统的HTTP协议不同,WebSocket协议是一种全双工的协议,并且比较轻量级。在通信时,客户端和服务器只需要完成一次握手,就可以建立起一条持久化的连接,然后任意一方都可以通过该连接发送消息给另一方。
在AndServer中,WebSocket的支持主要是通过添加WebSocket插件来实现。开发者只需要在项目中添加WebSocket插件的依赖,并在代码中添加相应的逻辑,就可以轻松地实现WebSocket通信。
WebSocket在实时通信方面具有很好的优势,因此在一些需要实时通信的应用场景中被广泛使用。例如在线聊天系统、实时游戏、股票行情等。同时,AndServer也提供了很好的支持,使得开发者能够更加轻松地实现WebSocket通信功能。
相关问题
C++ websocket server
你可以使用第三方库来实现 C++ 的 WebSocket 服务器,比如 Boost.Beast 和 WebSocket++。
使用 Boost.Beast 实现 WebSocket 服务器的基本步骤如下:
1. 创建一个 TCP 服务器并监听指定端口。
2. 接受来自客户端的连接请求。
3. 在接受到连接请求后,握手协议。
4. 在握手成功后,接收和发送 WebSocket 消息。
5. 处理错误和断开连接。
下面是一个使用 Boost.Beast 实现的简单的 WebSocket 服务器的示例代码:
```
#include <boost/beast.hpp>
#include <boost/asio.hpp>
#include <iostream>
#include <string>
namespace beast = boost::beast; // from <boost/beast.hpp>
namespace http = beast::http; // from <boost/beast/http.hpp>
namespace net = boost::asio; // from <boost/asio.hpp>
using tcp = net::ip::tcp; // from <boost/asio/ip/tcp.hpp>
int main()
{
try
{
net::io_context ioc;
tcp::acceptor acceptor(ioc, tcp::endpoint(tcp::v4(), 8080));
while (true)
{
tcp::socket socket(ioc);
acceptor.accept(socket);
// handshake
beast::flat_buffer buffer;
http::request<http::empty_body> req;
http::read(socket, buffer, req);
http::response<http::empty_body> res;
res.set(http::field::sec_websocket_accept, req["sec-websocket-key"] + "258EAFA5-E914-47DA-95CA-C5AB0DC85B11");
res.result(http::status::switching_protocols);
res.version(req.version());
http::write(socket, res);
// read and write websocket data
beast::websocket::stream<tcp::socket&> ws(socket);
ws.accept();
while (true)
{
beast::flat_buffer buffer;
ws.read(buffer);
std::string message(beast::buffers_to_string(buffer.data()));
if (message == "exit")
{
break;
}
ws.write(net::buffer(std::string("Server: ") + message));
}
ws.close(beast::websocket::close_code::normal);
}
}
catch (std::exception& e)
{
std::cerr << "Error: " << e.what() << std::endl;
return 1;
}
return 0;
}
```
使用 WebSocket++ 实现 WebSocket 服务器的基本步骤如下:
1. 创建一个服务器并设置回调函数。
2. 接受来自客户端的连接请求。
3. 在接受到连接请求后,握手协议。
4. 在握手成功后,接收和发送 WebSocket 消息。
5. 处理错误和断开连接。
下面是一个使用 WebSocket++ 实现的简单的 WebSocket 服务器的示例代码:
```
#include <websocketpp/config/asio_no_tls.hpp>
#include <websocketpp/server.hpp>
#include <iostream>
#include <string>
using websocketpp::lib::placeholders::_1;
using websocketpp::lib::placeholders::_2;
using websocketpp::lib::bind;
class Server
{
public:
Server()
{
server_.set_access_channels(websocketpp::log::alevel::none);
server_.clear_access_channels(websocketpp::log::alevel::none);
server_.set_message_handler(bind(&Server::on_message, this, _1, _2));
}
void run(uint16_t port)
{
server_.listen(port);
server_.start_accept();
server_.run();
}
private:
void on_message(websocketpp::connection_hdl hdl, websocketpp::server<websocketpp::config::asio>*, websocketpp::frame::opcode::value opcode, std::string message)
{
if (opcode == websocketpp::frame::opcode::text)
{
if (message == "exit")
{
server_.stop();
}
else
{
server_.send(hdl, "Server: " + message, opcode);
}
}
}
websocketpp::server<websocketpp::config::asio> server_;
};
int main()
{
try
{
Server server;
server.run(8080);
}
catch (websocketpp::exception const& e)
{
std::cerr << "Error: " << e.what() << std::endl;
return 1;
}
return 0;
}
```
WebSocketServer java.io.EOFException
java.io.EOFException is an exception thrown by java I/O when an end of file or end of stream has been reached unexpectedly during input. In the context of a WebSocketServer, this error could occur if the connection between the server and client is closed unexpectedly, such as when the client terminates the connection.
To troubleshoot this issue, you can try the following steps:
1. Check the logs to see if there are any error messages or stack traces that might provide more information about the cause of the exception.
2. Ensure that the client is not terminating the connection unexpectedly. You can do this by checking the client-side code and ensuring that it is properly closing the connection when it is no longer needed.
3. Check the server-side code to ensure that it is handling WebSocket connections correctly and not prematurely closing the connection.
4. Make sure that your WebSocket server implementation is compatible with the WebSocket protocol version used by the client.
If none of these steps resolve the issue, you may need to seek additional help or consult the documentation for your WebSocket server implementation to see if there are any known issues or troubleshooting steps specific to that implementation.
阅读全文
相关推荐
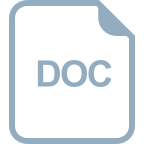
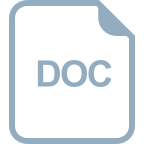
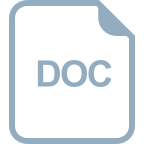
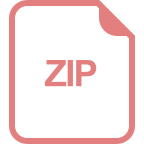
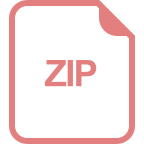
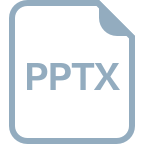
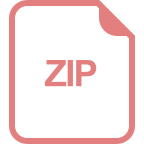
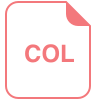








