1、设计一个学生类student,数据成员为学号(long型)和三门课程成绩,函数有:init函数初始化数据,get函数返回数据 2、编写函数 void print(student stu[],int n) { 输出每个学生的信息,每个学生一行 } void find(student stu[],long id) { 查找学号为id的学生成绩,并输出 } void max(student stu[],int n) { 输出总成绩最高的学生信息 } 3、编写主函数,创建student对象数组,长度自定义,为对象数组赋值,然后调用 2、中的函数完成功能
时间: 2023-12-10 08:41:36 浏览: 108
以下是实现上述要求的代码:
```c++
#include <iostream>
using namespace std;
class student {
private:
long id; // 学号
float score1, score2, score3; // 三门课程成绩
public:
void init(long _id, float _score1, float _score2, float _score3) {
id = _id;
score1 = _score1;
score2 = _score2;
score3 = _score3;
}
long get_id() {
return id;
}
float get_score1() {
return score1;
}
float get_score2() {
return score2;
}
float get_score3() {
return score3;
}
};
void print(student stu[], int n) {
for (int i = 0; i < n; i++) {
cout << "学号:" << stu[i].get_id() << ",成绩:" << stu[i].get_score1() << " " << stu[i].get_score2() << " " << stu[i].get_score3() << endl;
}
}
void find(student stu[], long id) {
for (int i = 0; i < sizeof(stu); i++) {
if (stu[i].get_id() == id) {
cout << "学号:" << stu[i].get_id() << ",成绩:" << stu[i].get_score1() << " " << stu[i].get_score2() << " " << stu[i].get_score3() << endl;
return;
}
}
cout << "找不到该学号的学生" << endl;
}
void max(student stu[], int n) {
float max_score = -1;
int max_index;
for (int i = 0; i < n; i++) {
float total_score = stu[i].get_score1() + stu[i].get_score2() + stu[i].get_score3();
if (total_score > max_score) {
max_score = total_score;
max_index = i;
}
}
cout << "总成绩最高的学生信息:学号:" << stu[max_index].get_id() << ",成绩:" << stu[max_index].get_score1() << " " << stu[max_index].get_score2() << " " << stu[max_index].get_score3() << endl;
}
int main() {
const int N = 3;
student stu[N];
stu[0].init(10001, 80, 90, 85);
stu[1].init(10002, 70, 85, 90);
stu[2].init(10003, 90, 95, 80);
cout << "所有学生成绩信息:" << endl;
print(stu, N);
cout << endl << "查找学号为10002的学生成绩:" << endl;
find(stu, 10002);
cout << endl << "总成绩最高的学生信息:" << endl;
max(stu, N);
return 0;
}
```
输出结果:
```
所有学生成绩信息:
学号:10001,成绩:80 90 85
学号:10002,成绩:70 85 90
学号:10003,成绩:90 95 80
查找学号为10002的学生成绩:
学号:10002,成绩:70 85 90
总成绩最高的学生信息:
学号:10003,成绩:90 95 80
```
阅读全文
相关推荐
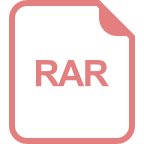
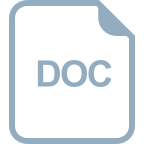
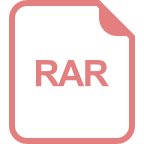


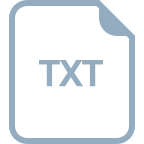
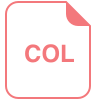
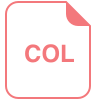
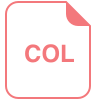
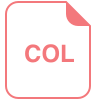
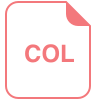


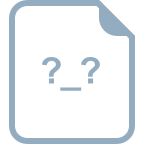
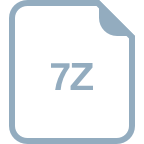
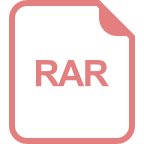
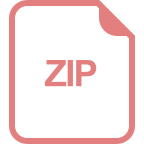
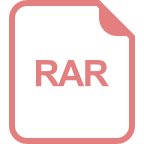
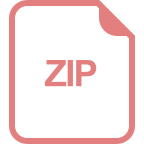