go-plugin example
时间: 2023-06-30 15:26:26 浏览: 57
Sure! Here's an example of how to use go-plugin:
First, you need to create a plugin. Here's an example plugin called "example_plugin.go":
```
package main
import "fmt"
type ExamplePlugin struct{}
func (p *ExamplePlugin) SayHello() {
fmt.Println("Hello from ExamplePlugin!")
}
var Plugin ExamplePlugin
```
This plugin defines a type called "ExamplePlugin" with a single method called "SayHello". When this method is called, it prints "Hello from ExamplePlugin!" to the console.
Next, you need to create a host program that loads the plugin. Here's an example host program called "example_host.go":
```
package main
import (
"fmt"
"log"
"github.com/hashicorp/go-plugin"
)
func main() {
pluginMap := map[string]plugin.Plugin{
"example_plugin": &ExamplePlugin{},
}
client := plugin.NewClient(&plugin.ClientConfig{
HandshakeConfig: plugin.HandshakeConfig{
ProtocolVersion: 1,
MagicCookieKey: "EXAMPLE_PLUGIN",
MagicCookieValue: "1234",
},
Plugins: pluginMap,
})
defer client.Kill()
raw, err := client.Dispense("example_plugin")
if err != nil {
log.Fatal(err)
}
examplePlugin, ok := raw.(*ExamplePlugin)
if !ok {
log.Fatal("Failed to cast plugin to ExamplePlugin")
}
examplePlugin.SayHello()
}
```
This host program creates a plugin map that maps the name "example_plugin" to an instance of the ExamplePlugin type defined in the plugin. It then creates a client that connects to the plugin using the HandshakeConfig and plugin map, and retrieves an instance of the ExamplePlugin type using the client's Dispense function. Finally, it calls the SayHello method on the ExamplePlugin instance.
To run this example, you would first need to build the plugin by running "go build -buildmode=plugin example_plugin.go". Then you would run the host program by running "go run example_host.go". This should output "Hello from ExamplePlugin!" to the console.
相关推荐
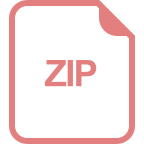















