用Spring AOP技术实现对用户的删除,添加,修改,查询操作按如下格式,[调用的那个类,哪个方法,调用时间,执行的操作(增,删,改,查)]等信息,通过java的io操作实现将日志记录在log.txt文件中
时间: 2023-05-12 11:05:11 浏览: 113
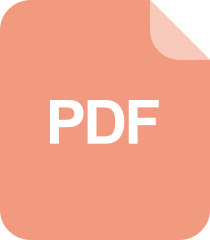
spring aop之链式调用的实现
可以使用Spring AOP的@Aspect注解来实现对用户的删除、添加、修改、查询操作的日志记录。具体实现步骤如下:
1. 定义一个切面类,使用@Aspect注解标注,并在类中定义切点和通知方法。
2. 在通知方法中,使用JoinPoint对象获取调用的类、方法、参数等信息,并将这些信息组合成日志信息,最后通过Java的IO操作将日志记录在log.txt文件中。
下面是一个简单的实现示例:
@Aspect
public class UserLogAspect {
private static final Logger logger = LoggerFactory.getLogger(UserLogAspect.class);
@Pointcut("execution(* com.example.UserService.*(..))")
public void userPointcut() {}
@Before("userPointcut()")
public void beforeUser(JoinPoint joinPoint) {
String methodName = joinPoint.getSignature().getName();
Object[] args = joinPoint.getArgs();
String operation = "";
if (methodName.startsWith("add")) {
operation = "添加";
} else if (methodName.startsWith("delete")) {
operation = "删除";
} else if (methodName.startsWith("update")) {
operation = "修改";
} else if (methodName.startsWith("get")) {
operation = "查询";
}
String logMsg = String.format("调用类:%s,方法:%s,调用时间:%s,执行操作:%s",
joinPoint.getTarget().getClass().getName(), methodName, new Date(), operation);
logger.info(logMsg);
}
}
在上面的代码中,我们定义了一个切面类UserLogAspect,并在其中定义了一个切点userPointcut,用于匹配UserService类中的所有方法。
然后,我们在通知方法beforeUser中使用JoinPoint对象获取调用的类、方法、参数等信息,并将这些信息组合成日志信息,最后通过LoggerFactory.getLogger方法获取Logger对象,使用logger.info方法将日志记录在log.txt文件中。
需要注意的是,上面的代码中使用了Spring的日志框架Logback,需要在项目中添加相关依赖。
至此,我们就成功地使用Spring AOP技术实现了对用户的删除、添加、修改、查询操作的日志记录。
阅读全文
相关推荐
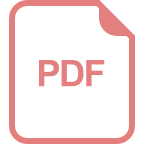
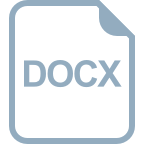














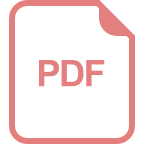
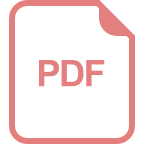