使用python和sqlsever写一个客户信息管理系统的代码
时间: 2024-02-15 18:03:36 浏览: 108
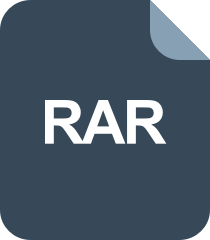
python+SQLsever+tkinter学生宿舍管理系统

好的,以下是一个使用Python和SQL Server实现的简单客户信息管理系统的代码示例,仅供参考:
```python
import pyodbc
# 连接SQL Server数据库
conn = pyodbc.connect('DRIVER={SQL Server};SERVER=localhost;DATABASE=mydb;UID=username;PWD=password')
# 客户信息表格
customers_table = 'customers'
# 客户信息管理系统
class CustomerManagementSystem:
def __init__(self):
pass
# 添加客户信息
def add_customer(self, name, email, phone):
cursor = conn.cursor()
cursor.execute(f"INSERT INTO {customers_table} (name, email, phone) VALUES (?, ?, ?)", name, email, phone)
conn.commit()
cursor.close()
# 查询客户信息
def get_customer(self, id):
cursor = conn.cursor()
cursor.execute(f"SELECT * FROM {customers_table} WHERE id=?", id)
row = cursor.fetchone()
cursor.close()
return row
# 更新客户信息
def update_customer(self, id, name=None, email=None, phone=None):
cursor = conn.cursor()
if name:
cursor.execute(f"UPDATE {customers_table} SET name=? WHERE id=?", name, id)
if email:
cursor.execute(f"UPDATE {customers_table} SET email=? WHERE id=?", email, id)
if phone:
cursor.execute(f"UPDATE {customers_table} SET phone=? WHERE id=?", phone, id)
conn.commit()
cursor.close()
# 删除客户信息
def delete_customer(self, id):
cursor = conn.cursor()
cursor.execute(f"DELETE FROM {customers_table} WHERE id=?", id)
conn.commit()
cursor.close()
# 查询所有客户信息
def get_all_customers(self):
cursor = conn.cursor()
cursor.execute(f"SELECT * FROM {customers_table}")
rows = cursor.fetchall()
cursor.close()
return rows
# 客户信息管理系统示例
if __name__ == '__main__':
cms = CustomerManagementSystem()
cms.add_customer('张三', 'zs@example.com', '1234567890')
cms.add_customer('李四', 'ls@example.com', '0987654321')
cms.update_customer(1, name='王五')
cms.delete_customer(2)
customers = cms.get_all_customers()
for customer in customers:
print(customer)
```
这个代码示例实现了一个简单的客户信息管理系统,包括了添加客户信息、查询客户信息、更新客户信息、删除客户信息和查询所有客户信息等功能。也可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐
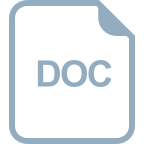
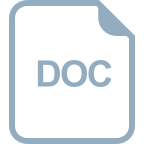

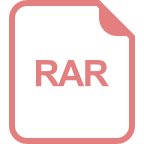







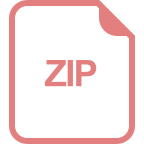
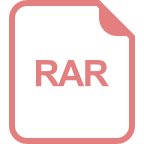




