在使用word2vec进行文本特征提取时,如何将缺失的单词向量替换为一个默认向量,或者完全忽略缺失的单词
时间: 2024-03-05 07:55:17 浏览: 123
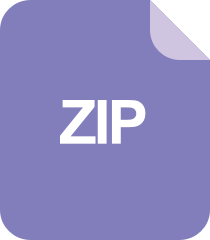
词向量-嵌入word2vec词向量的cnn中文文本分类.zip
在使用 word2vec 进行文本特征提取时,如果某些单词在训练数据中没有出现过,那么它们的词向量是缺失的。可以考虑使用以下两种方法来处理这种情况:
1. 将缺失的单词向量替换为一个默认向量:可以使用一个固定的默认向量来替换所有缺失的单词向量。这个默认向量可以选择为全零向量、随机向量或者其他合适的向量。具体实现时,可以在训练模型之前,将所有缺失的单词向量替换为默认向量,然后再进行训练。
下面是一个使用全零向量来替换缺失单词向量的示例代码:
```python
import numpy as np
from gensim.models import Word2Vec
# 训练数据
sentences = [['i', 'like', 'apple'], ['he', 'likes', 'banana'], ['she', 'likes', 'orange']]
# 训练 word2vec 模型
model = Word2Vec(sentences, size=3, window=5, min_count=1, workers=4)
# 获取所有单词的词向量
vocab = list(model.wv.vocab)
word_vectors = []
for word in vocab:
word_vectors.append(model.wv[word])
word_vectors = np.array(word_vectors)
# 将缺失的单词向量替换为全零向量
default_vector = np.zeros(3)
default_idx = vocab.index('<DEFAULT>')
for i in range(len(word_vectors)):
if np.all(word_vectors[i] == 0):
word_vectors[i] = default_vector
vocab[i] = '<DEFAULT>'
# 训练模型
model.train(sentences, total_examples=len(sentences), epochs=100)
# 获取单词向量
print(model.wv[vocab])
```
2. 完全忽略缺失的单词:如果某个单词在训练数据中没有出现过,也没有提前设定默认向量,那么可以选择完全忽略它,不将其包含在训练数据中。具体实现时,可以在训练模型之前,将所有缺失的单词从训练数据中剔除,然后再进行训练。
下面是一个忽略缺失单词的示例代码:
```python
from gensim.models import Word2Vec
# 训练数据
sentences = [['i', 'like', 'apple'], ['he', 'likes', 'banana'], ['she', 'likes', 'orange']]
# 获取所有单词
vocab = set()
for sentence in sentences:
for word in sentence:
vocab.add(word)
# 移除缺失的单词
missing_words = set()
for word in vocab:
if word not in model.wv.vocab:
missing_words.add(word)
for sentence in sentences:
for word in sentence:
if word in missing_words:
sentence.remove(word)
# 训练模型
model = Word2Vec(sentences, size=3, window=5, min_count=1, workers=4)
# 获取单词向量
print(model.wv[list(vocab)])
```
在实际应用中,需要根据具体的场景,选择合适的处理方法。如果缺失的单词对于模型的性能影响较小,可以选择使用默认向量或忽略缺失单词;如果缺失的单词对于模型的性能影响较大,需要考虑其他更加有效的补充方法。
阅读全文
相关推荐
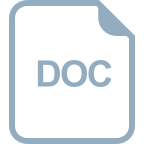
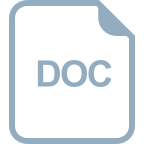
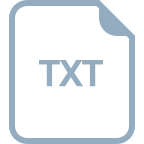
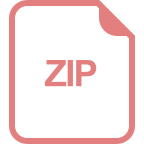
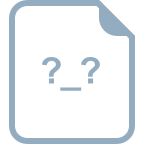
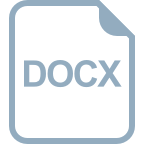
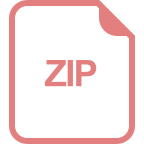
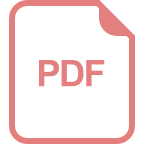
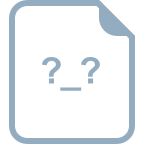
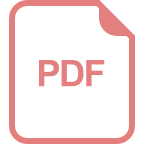
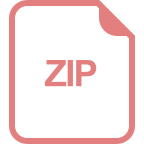
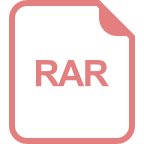
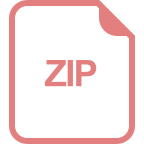
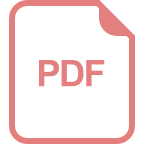

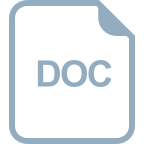