用c语言设计一个一元稀疏多项式简单乘法计算器
时间: 2023-05-17 16:06:59 浏览: 72
好的,以下是一个简单的一元稀疏多项式简单乘法计算器的C语言代码:
```
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int coef;
int exp;
struct node *next;
} Node;
Node *createNode(int coef, int exp) {
Node *newNode = (Node*)malloc(sizeof(Node));
newNode->coef = coef;
newNode->exp = exp;
newNode->next = NULL;
return newNode;
}
void insertNode(Node **head, int coef, int exp) {
Node *newNode = createNode(coef, exp);
if (*head == NULL) {
*head = newNode;
} else {
Node *cur = *head;
while (cur->next != NULL) {
cur = cur->next;
}
cur->next = newNode;
}
}
void printList(Node *head) {
Node *cur = head;
while (cur != NULL) {
printf("%dx^%d ", cur->coef, cur->exp);
cur = cur->next;
}
printf("\n");
}
Node *multiply(Node *poly1, Node *poly2) {
Node *result = NULL;
Node *cur1 = poly1;
while (cur1 != NULL) {
Node *cur2 = poly2;
while (cur2 != NULL) {
int coef = cur1->coef * cur2->coef;
int exp = cur1->exp + cur2->exp;
insertNode(&result, coef, exp);
cur2 = cur2->next;
}
cur1 = cur1->next;
}
return result;
}
int main() {
Node *poly1 = NULL;
Node *poly2 = NULL;
insertNode(&poly1, 2, 3);
insertNode(&poly1, 3, 2);
insertNode(&poly1, 4, 1);
insertNode(&poly1, 5, 0);
insertNode(&poly2, 1, 2);
insertNode(&poly2, 2, 1);
insertNode(&poly2, 3, 0);
printf("Poly1: ");
printList(poly1);
printf("Poly2: ");
printList(poly2);
Node *result = multiply(poly1, poly2);
printf("Result: ");
printList(result);
return 0;
}
```
这个程序可以接受两个多项式作为输入,然后计算它们的乘积并输出结果。
相关推荐
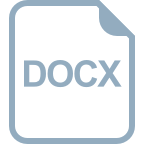
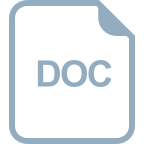














