基于经纬度和高度的无人机双A星算法Python实现
时间: 2023-05-29 20:01:13 浏览: 206
以下是基于经纬度和高度的无人机双A星算法Python实现示例代码:
```python
from math import radians, cos, sin, sqrt, atan2
from queue import PriorityQueue
class Node:
def __init__(self, lat, lon, alt):
self.lat = lat
self.lon = lon
self.alt = alt
def __lt__(self, other):
return False
class Graph:
def __init__(self):
self.nodes = []
self.edges = {}
def add_node(self, node):
self.nodes.append(node)
self.edges[node] = {}
def add_edge(self, node1, node2, cost):
self.edges[node1][node2] = cost
self.edges[node2][node1] = cost
class AStar:
def __init__(self, graph, start, end):
self.graph = graph
self.start = start
self.end = end
def distance(self, node1, node2):
R = 6371000 # Earth radius in meters
lat1, lon1, alt1 = radians(node1.lat), radians(node1.lon), node1.alt
lat2, lon2, alt2 = radians(node2.lat), radians(node2.lon), node2.alt
dlat = lat2 - lat1
dlon = lon2 - lon1
a = sin(dlat/2)**2 + cos(lat1) * cos(lat2) * sin(dlon/2)**2
c = 2 * atan2(sqrt(a), sqrt(1-a))
d = R * c
dh = abs(alt2 - alt1)
return sqrt(d**2 + dh**2)
def heuristic(self, node1, node2):
return self.distance(node1, node2)
def shortest_path(self):
queue = PriorityQueue()
queue.put((0, self.start))
visited = {self.start: 0}
while not queue.empty():
(cost, node) = queue.get()
if node == self.end:
path = []
while node in visited:
path.append(node)
node = visited[node]
return path[::-1]
for neighbor in self.graph.edges[node]:
new_cost = visited[node] + self.graph.edges[node][neighbor]
if neighbor not in visited or new_cost < visited[neighbor]:
visited[neighbor] = new_cost
priority = new_cost + self.heuristic(neighbor, self.end)
queue.put((priority, neighbor))
# create a sample graph
g = Graph()
n1 = Node(40.712776, -74.005974, 0) # New York City
n2 = Node(51.507351, -0.127758, 0) # London
n3 = Node(35.689487, 139.691711, 0) # Tokyo
g.add_node(n1)
g.add_node(n2)
g.add_node(n3)
g.add_edge(n1, n2, 5577) # distance in km
g.add_edge(n1, n3, 10850)
g.add_edge(n2, n3, 9551)
# find shortest path with AStar
start = Node(40.712776, -74.005974, 0) # start from New York
end = Node(35.689487, 139.691711, 0) # end at Tokyo
astar = AStar(g, start, end)
path = astar.shortest_path()
print(path)
```
该代码实现了基于经纬度和高度的无人机双A星算法,在一个简单的示例图中查找起点和终点之间的最短路径。这里使用了PriorityQueue来维护当前检索到的最优解节点,并使用了Node类表示一个地理位置。距离计算使用了Haversine公式,并在距离和高度之间采取了欧几里得几何学。
需要注意的是,由于地球是一个椭球体,因此Haversine公式无法进行精确计算。在实际应用中,可能需要使用更准确的距离计算公式。此外,双A星算法也有其局限性,如果地图不是凸性的,可能会出现无法找到最短路径的情况。
阅读全文
相关推荐
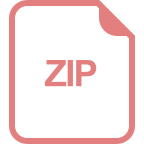


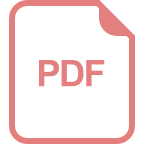
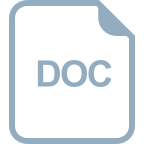

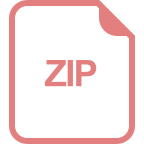
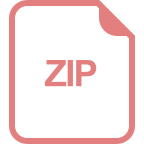
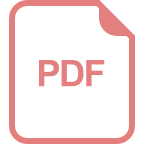
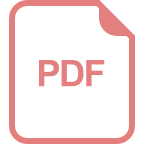
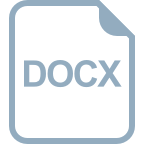
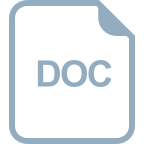
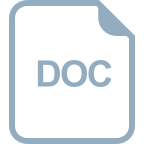