linux 共享内存跨进程传递 例子
时间: 2023-10-12 08:15:37 浏览: 67
下面是一个简单的 Linux 共享内存跨进程传递的例子:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#define SHM_KEY 1234
#define SHM_SIZE 1024
int main()
{
// 创建共享内存
int shmid = shmget(SHM_KEY, SHM_SIZE, IPC_CREAT | 0666);
if (shmid == -1) {
perror("shmget");
exit(1);
}
// 连接共享内存
char *shmaddr = shmat(shmid, NULL, 0);
if (shmaddr == (void *)-1) {
perror("shmat");
exit(1);
}
// 写入共享内存
strcpy(shmaddr, "Hello, world!");
// 分离共享内存
shmdt(shmaddr);
// 创建子进程
pid_t pid = fork();
if (pid == -1) {
perror("fork");
exit(1);
} else if (pid == 0) {
// 子进程连接共享内存
char *shmaddr = shmat(shmid, NULL, 0);
if (shmaddr == (void *)-1) {
perror("shmat");
exit(1);
}
// 读取共享内存
printf("Child process read: %s\n", shmaddr);
// 分离共享内存
shmdt(shmaddr);
// 删除共享内存
shmctl(shmid, IPC_RMID, NULL);
exit(0);
} else {
// 等待子进程结束
wait(NULL);
}
return 0;
}
```
这个例子中创建了一个大小为 1024 的共享内存,然后在主进程中写入了一段字符串 "Hello, world!",接着创建了一个子进程,子进程连接到共享内存并读取其中的内容,最后删除了这个共享内存。注意,这里使用了 IPC_RMID 命令将共享内存删除,这是因为一旦所有连接到该共享内存的进程都将其分离(使用 shmdt),那么该共享内存就会被删除,这样可以避免共享内存占用过多系统资源。
相关推荐
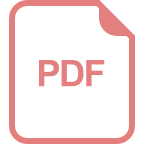
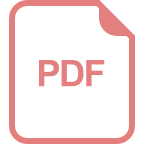
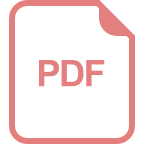














