能提供一个详细的Python代码示例,用于YOLO遥感数据集上的水平翻转、缩放和随机裁剪操作吗?同时,如何对应地更新txt标签文件,并且整个过程应支持将处理后的图像和标签保存到新的文件夹中?
时间: 2024-10-22 10:16:36 浏览: 23
当然,我可以为你提供一个基本的Python代码示例,使用OpenCV库来对遥感数据集(如YOLO数据集)进行水平翻转、缩放和随机裁剪操作。这里假设你已经有了一个`img`数组代表图像,`labels`是一个包含每个框坐标和类别的txt文件的路径。
```python
import cv2
import numpy as np
from PIL import Image
# 定义图像处理函数
def preprocess_image(img_path, labels_path, output_dir, width=None, height=None, flip=False, crop=False, scale=1.0):
# 打开图片
img = cv2.imread(img_path)
# 如果指定宽度和高度,进行缩放
if width and height:
img = cv2.resize(img, None, fx=scale, fy=scale, interpolation=cv2.INTER_LINEAR)
# 水平翻转
if flip:
img = cv2.flip(img, 1) # 1代表沿着x轴翻转
# 随机裁剪
if crop:
h, w, _ = img.shape
x = np.random.randint(0, w - width)
y = np.random.randint(0, h - height)
img = img[y:y+height, x:x+width]
# 保存图片到新目录
new_img_name = os.path.basename(img_path).split('.')[0] + '_processed.jpg'
output_img_path = os.path.join(output_dir, new_img_name)
cv2.imwrite(output_img_path, img)
# 更新txt标签文件
with open(labels_path, 'r') as f, open(os.path.join(output_dir, 'labels.txt'), 'w') as f_new:
for line in f:
# 提取坐标信息
coords = line.strip().split(',')
# 根据坐标调整
if crop:
left, top, right, bottom = int(coords[1]) * scale, int(coords[2]) * scale, int(coords[3]) * scale, int(coords[4]) * scale
else:
left, top, right, bottom = int(coords[1]), int(coords[2]), int(coords[3]), int(coords[4])
# 写入新文件
new_line = '{},{},{},{},{}\n'.format(left+x, top+y, right+x, bottom+y, coords[-1])
f_new.write(new_line)
# 示例用法
preprocess_image('path_to_your_image.jpg', 'path_to_your_labels.txt', 'output_directory', width=640, height=480, flip=True, crop=True)
```
这个代码会首先读取原始图片,然后按需求进行缩放、翻转和随机裁剪。对于每个框,根据操作调整其坐标并在新的txt文件中记录下来。最后,图片会被保存到指定的输出目录。
请注意,此代码简化了处理流程,实际应用中你可能还需要考虑更多细节,如文件路径管理、错误处理等。
阅读全文
相关推荐
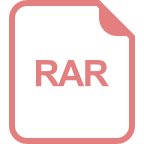
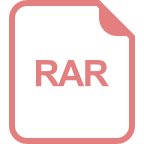
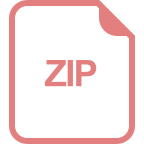

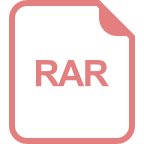
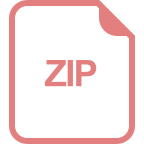
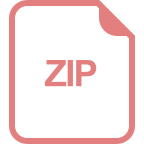
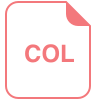
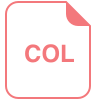
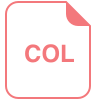
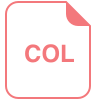
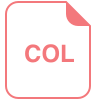
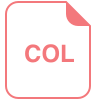
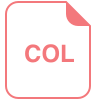
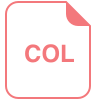
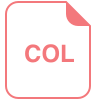
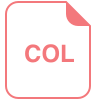
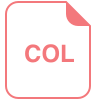