用c++语言编写的文件管理程序代码
时间: 2024-02-20 22:02:21 浏览: 26
以下是一个简单的使用C++实现文件管理的示例程序。该程序可以列出指定目录下的所有文件和子目录,并支持文件的复制、剪切、删除等基本操作。
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <dirent.h>
#include <unistd.h>
using namespace std;
const string ROOT_PATH = "/"; // 根目录路径
// 列出指定目录下的所有文件和子目录
vector<string> list_directory(const string& path) {
vector<string> files;
DIR* dir = opendir(path.c_str());
if (dir != nullptr) {
struct dirent* entry;
while ((entry = readdir(dir)) != nullptr) {
string name = entry->d_name;
if (name != "." && name != "..") {
string full_path = path + "/" + name;
files.push_back(full_path);
}
}
closedir(dir);
}
return files;
}
// 复制文件或目录
bool copy_file(const string& src_path, const string& dst_path) {
ifstream src(src_path, ios::binary);
ofstream dst(dst_path, ios::binary);
if (src && dst) {
dst << src.rdbuf();
return true;
}
return false;
}
// 剪切文件或目录
bool move_file(const string& src_path, const string& dst_path) {
if (rename(src_path.c_str(), dst_path.c_str()) == 0) {
return true;
}
return false;
}
// 删除文件或目录
bool delete_file(const string& path) {
if (remove(path.c_str()) == 0) {
return true;
}
return false;
}
int main() {
string current_path = ROOT_PATH; // 当前目录路径
vector<string> files = list_directory(current_path);
while (true) {
// 显示当前目录下的所有文件和子目录
cout << "Current directory: " << current_path << endl;
for (int i = 0; i < files.size(); i++) {
string name = files[i].substr(current_path.length() + 1);
cout << (i + 1) << ". " << name << endl;
}
// 读取用户输入的命令
cout << "Enter command (ls/cp/mv/rm/cd/exit): ";
string command;
cin >> command;
// 处理命令
if (command == "ls") { // 列出文件和子目录
files = list_directory(current_path);
} else if (command == "cp") { // 复制文件或目录
string src_path, dst_path;
cout << "Enter source path: ";
cin >> src_path;
cout << "Enter destination path: ";
cin >> dst_path;
if (copy_file(src_path, dst_path)) {
cout << "Copy succeeded!" << endl;
} else {
cout << "Copy failed!" << endl;
}
} else if (command == "mv") { // 剪切文件或目录
string src_path, dst_path;
cout << "Enter source path: ";
cin >> src_path;
cout << "Enter destination path: ";
cin >> dst_path;
if (move_file(src_path, dst_path)) {
cout << "Move succeeded!" << endl;
} else {
cout << "Move failed!" << endl;
}
} else if (command == "rm") { // 删除文件或目录
string path;
cout << "Enter path: ";
cin >> path;
if (delete_file(path)) {
cout << "Delete succeeded!" << endl;
} else {
cout << "Delete failed!" << endl;
}
} else if (command == "cd") { // 切换目录
string path;
cout << "Enter path: ";
cin >> path;
if (chdir(path.c_str()) == 0) {
current_path = path;
files = list_directory(current_path);
} else {
cout << "Change directory failed!" << endl;
}
} else if (command == "exit") { // 退出程序
break;
} else {
cout << "Invalid command!" << endl;
}
}
return 0;
}
```
请注意,这只是一个简单的示例程序,实际应用中还需要考虑更多的细节和安全性问题。例如,需要检查文件是否存在、是否具有读写权限等。同时,需要对用户输入进行验证和过滤,以避免恶意操作和安全漏洞。
相关推荐
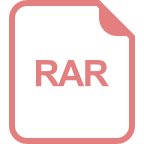
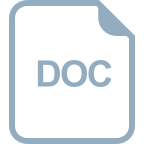














