帮我写一个编译C++程序的函数并把函数提取到TXT文件中的程序
时间: 2023-08-31 13:20:44 浏览: 61
### 回答1:
我可以为你编写一个程序,用于将编译C程序的函数提取到TXT文件中。代码如下:#include <stdio.h>
#include <stdlib.h>// 函数声明
void extractFunction (char *fileName, char *functionName);// 主函数
int main (int argc, char *argv[])
{
// 检查输入参数
if (argc < 3)
{
printf("Usage: extractFunction <fileName> <functionName>\n");
return 1;
}
// 提取函数
extractFunction(argv[1], argv[2]);
return 0;
}// 提取函数
void extractFunction (char *fileName, char *functionName)
{
FILE *fp;
char line[200];
// 打开文件
fp = fopen(fileName, "r");
if (fp == NULL)
{
perror("Error opening file");
return;
}
// 读取文件中的每一行
while (fgets(line, 200, fp) != NULL)
{
// 检查该行是否为提取的函数
if (strstr(line, functionName) != NULL)
{
// 如果是,则将该行写入TXT文件
FILE *fp2 = fopen("output.txt", "w");
if (fp2 == NULL)
{
perror("Error opening file");
return;
}
fprintf(fp2, "%s", line);
fclose(fp2);
}
}
fclose(fp);
}
### 回答2:
下面是一个示例程序,用于编译C程序并将函数提取到TXT文件中:
```c
#include <stdio.h>
#include <stdlib.h>
// 编译C程序并提取函数到TXT文件中的函数
void compileAndExtractFunction(const char* filename, const char* functionName) {
// 构建编译命令
char command[100] = "gcc -o temp ";
strcat(command, filename);
strcat(command, " -lm");
// 执行编译命令
system(command);
// 构建提取函数命令
char extractFunctionCommand[100] = "objdump -d temp | grep -A20 \"<";
strcat(extractFunctionCommand, functionName);
strcat(extractFunctionCommand, ">\" > function.txt");
// 执行提取函数命令
system(extractFunctionCommand);
printf("函数提取完成!请查看function.txt文件。\n");
}
int main() {
// 输入C文件名和函数名
const char* filename = "example.c";
const char* functionName = "myFunction";
// 调用函数进行编译和提取
compileAndExtractFunction(filename, functionName);
return 0;
}
```
上述程序中,`compileAndExtractFunction`函数用于编译C程序并把函数提取到`function.txt`文件中。在`main`函数中,你可以设置要编译的C文件名和需要提取的函数名。
请注意,该程序需要依赖GCC编译器和objdump工具。在Linux系统中,这些工具通常已经安装。在Windows系统中,你需要确保已安装MinGW或Cygwin等工具,并将它们的安装路径添加到环境变量中。
编译并运行程序后,函数提取结果将保存在`function.txt`文件中。你可以按需修改文件名和路径来满足你的实际需求。
### 回答3:
下面是一个编译C程序并将函数提取到TXT文件中的程序示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
void compileCProgram(const char* programPath, const char* outputPath)
{
// 构建编译命令
char command[100];
sprintf(command, "gcc %s -o %s", programPath, outputPath);
// 执行编译命令
system(command);
// 打开输出文件
FILE* outputFile = fopen(outputPath, "a");
// 判断是否成功生成可执行文件
if (outputFile == NULL) {
printf("编译失败!\n");
return;
}
// 打开源代码文件
FILE* programFile = fopen(programPath, "r");
// 判断是否成功打开源程序文件
if (programFile == NULL) {
printf("无法打开源程序文件!\n");
fclose(outputFile);
return;
}
// 逐行读取源代码文件内容并写入到TXT文件中
char line[100];
while (fgets(line, sizeof(line), programFile) != NULL) {
fputs(line, outputFile);
}
// 关闭文件
fclose(programFile);
fclose(outputFile);
printf("编译成功!生成的TXT文件路径:%s\n", outputPath);
}
int main()
{
const char* programPath = "program.c"; // 源代码文件路径
const char* outputPath = "output.txt"; // 输出TXT文件路径
compileCProgram(programPath, outputPath);
return 0;
}
```
该程序通过调用gcc命令使用系统函数system进行C程序的编译。编译成功后,再使用文件读写函数将源代码内容逐行写入到TXT文件中。通过在main函数中调用compileCProgram函数,可以将指定的源代码文件编译并将其处理结果写入到指定的TXT文件中。
相关推荐
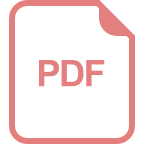
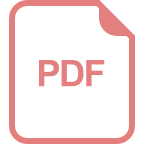














