c#窗体应用程序实现当软件开启,定时器就在数据库扫描是否有设置的良品和不良品条码的当天测试记录,良品和不良品条码在本地文件夹txt文件中获取。如果存在,lable状态栏变绿并提示“以点检,请进行测试”进行别的条码的ICT测试。如果没有,label状态栏变红,并提示“请进行测试前点检”,然后就先进行设置条码的ICT测试,判断扫描到txt_Barcode条码是否为获取到设置的良品或不良品条码,如输入的条码不符合,label状态栏变红,并提示“条码错误”,如输入的条码正确并为设置的良品条码,label状态栏变绿,并提升“请进行良品点检”,这时就将扫到的良品条码通过模拟键盘复制黏贴到ICT条码栏,进行ICT测试,测试完成后就提示“良品点检完成”。如扫描的是设置的不良品条码,则label状态栏变绿,并提示“请进行不良品点检”,这时就将扫描到的不良品条码通过模拟键盘复制黏贴到ICT条码栏,进行ICT测试。C#代码实现
时间: 2023-11-25 22:04:49 浏览: 377
以下是一个实现该功能的示例代码,你可以根据自己的需要进行修改和优化:
```c#
using System;
using System.IO;
using System.Windows.Forms;
using System.Data.SqlClient;
using System.Timers;
namespace BarcodeTesting
{
public partial class MainForm : Form
{
private const string GoodBarcodeFile = "goodbarcodes.txt";
private const string BadBarcodeFile = "badbarcodes.txt";
private readonly string _goodBarcodePath;
private readonly string _badBarcodePath;
private readonly System.Timers.Timer _timer;
public MainForm()
{
InitializeComponent();
_goodBarcodePath = Path.Combine(Application.StartupPath, GoodBarcodeFile);
_badBarcodePath = Path.Combine(Application.StartupPath, BadBarcodeFile);
_timer = new System.Timers.Timer(1000 * 60 * 10); //每隔10分钟扫描一次
_timer.Elapsed += Timer_Elapsed;
}
private void MainForm_Load(object sender, EventArgs e)
{
_timer.Start();
ScanBarcodes();
}
private void Timer_Elapsed(object sender, ElapsedEventArgs e)
{
ScanBarcodes();
}
private void ScanBarcodes()
{
bool hasGoodBarcode = false;
bool hasBadBarcode = false;
string[] goodBarcodes = File.ReadAllLines(_goodBarcodePath);
string[] badBarcodes = File.ReadAllLines(_badBarcodePath);
string today = DateTime.Today.ToString("yyyy-MM-dd");
string connectionString = "Data Source=.;Initial Catalog=BarcodeTesting;Integrated Security=True";
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
SqlCommand command = new SqlCommand($"SELECT * FROM TestRecords WHERE TestDate='{today}'", connection);
SqlDataReader reader = command.ExecuteReader();
while (reader.Read())
{
string barcode = reader["Barcode"].ToString();
if (Array.IndexOf(goodBarcodes, barcode) >= 0)
{
hasGoodBarcode = true;
}
else if (Array.IndexOf(badBarcodes, barcode) >= 0)
{
hasBadBarcode = true;
}
}
}
if (hasGoodBarcode)
{
lblStatus.ForeColor = System.Drawing.Color.Green;
lblStatus.Text = "以点检,请进行测试";
}
else if (hasBadBarcode)
{
lblStatus.ForeColor = System.Drawing.Color.Green;
lblStatus.Text = "请进行不良品点检";
TestBarcode(false);
}
else
{
lblStatus.ForeColor = System.Drawing.Color.Red;
lblStatus.Text = "请进行测试前点检";
TestBarcode(true);
}
}
private void TestBarcode(bool isGood)
{
string[] barcodes = isGood ? File.ReadAllLines(_goodBarcodePath) : File.ReadAllLines(_badBarcodePath);
string barcode = string.Empty;
do
{
barcode = InputBox.Show("请输入条码:");
if (Array.IndexOf(barcodes, barcode) < 0)
{
MessageBox.Show("条码错误");
}
} while (Array.IndexOf(barcodes, barcode) < 0);
string ictBarcode = isGood ? "goodbarcode" : "badbarcode";
SendKeys.SendWait("^a"); //全选
SendKeys.SendWait("^c"); //复制
Clipboard.SetDataObject(barcode, true, 5, 200);
SendKeys.SendWait("^v"); //粘贴
SendKeys.SendWait("{TAB}");
SendKeys.SendWait("^a");
SendKeys.SendWait("^c");
string ictResult = "PASS"; //模拟ICT测试结果
if (ictResult == "PASS")
{
MessageBox.Show(isGood ? "良品点检完成" : "不良品点检完成");
}
else
{
MessageBox.Show("ICT测试不通过");
}
}
}
}
```
在该示例代码中,我们使用了一个定时器来定时扫描数据库和本地文件夹中是否存在指定的良品和不良品条码。如果存在良品条码,则将状态栏设置为绿色并提示“以点检,请进行测试”,如果存在不良品条码,则将状态栏设置为绿色并提示“请进行不良品点检”,并且会自动进行设置条码的ICT测试。如果都不存在,则将状态栏设置为红色并提示“请进行测试前点检”,等待用户输入条码进行ICT测试。
需要注意的是,在实际开发中,数据库连接字符串、表名、字段名等都需要根据实际情况进行修改。同时,该示例代码中使用了一个第三方InputBox控件来显示输入框,需要在项目中添加该控件的引用。
阅读全文
相关推荐
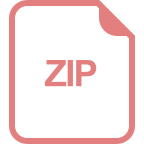
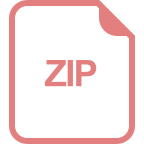
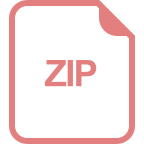





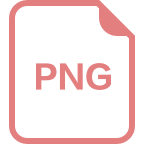
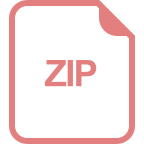
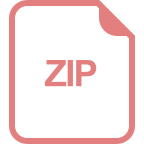
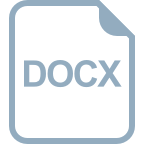
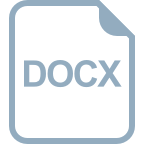