使用idea和mysql编写一个图书管理系统,主要功能为增删改查
时间: 2023-06-25 09:05:31 浏览: 213
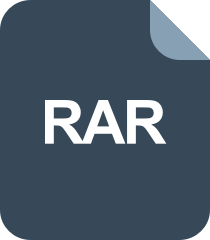
基于java+swing+mysql图书管理系统(简单的增删改查)
好的,下面是一个简单的图书管理系统的示例代码,使用Java语言,使用IDEA开发工具和MySQL数据库。
首先,创建一个名为Book的Java类,用于存储图书信息。
```java
public class Book {
private int id;
private String name;
private String author;
private double price;
public Book(int id, String name, String author, double price) {
this.id = id;
this.name = name;
this.author = author;
this.price = price;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public String getAuthor() {
return author;
}
public double getPrice() {
return price;
}
}
```
然后,创建一个名为BookDao的Java类,用于实现与MySQL数据库的交互。
```java
import java.sql.*;
import java.util.ArrayList;
import java.util.List;
public class BookDao {
private static final String URL = "jdbc:mysql://localhost:3306/bookstore";
private static final String USERNAME = "root";
private static final String PASSWORD = "123456";
public List<Book> getAllBooks() {
List<Book> books = new ArrayList<>();
try (Connection connection = DriverManager.getConnection(URL, USERNAME, PASSWORD);
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery("SELECT * FROM books")) {
while (resultSet.next()) {
int id = resultSet.getInt("id");
String name = resultSet.getString("name");
String author = resultSet.getString("author");
double price = resultSet.getDouble("price");
Book book = new Book(id, name, author, price);
books.add(book);
}
} catch (SQLException e) {
e.printStackTrace();
}
return books;
}
public void addBook(Book book) {
try (Connection connection = DriverManager.getConnection(URL, USERNAME, PASSWORD);
PreparedStatement statement = connection.prepareStatement("INSERT INTO books (name, author, price) VALUES (?, ?, ?)")) {
statement.setString(1, book.getName());
statement.setString(2, book.getAuthor());
statement.setDouble(3, book.getPrice());
statement.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
public void updateBook(Book book) {
try (Connection connection = DriverManager.getConnection(URL, USERNAME, PASSWORD);
PreparedStatement statement = connection.prepareStatement("UPDATE books SET name = ?, author = ?, price = ? WHERE id = ?")) {
statement.setString(1, book.getName());
statement.setString(2, book.getAuthor());
statement.setDouble(3, book.getPrice());
statement.setInt(4, book.getId());
statement.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
public void deleteBook(int id) {
try (Connection connection = DriverManager.getConnection(URL, USERNAME, PASSWORD);
PreparedStatement statement = connection.prepareStatement("DELETE FROM books WHERE id = ?")) {
statement.setInt(1, id);
statement.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
```
最后,创建一个名为Main的Java类,用于实现图书管理系统的主要功能。
```java
import java.util.List;
import java.util.Scanner;
public class Main {
private static final BookDao bookDao = new BookDao();
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("请选择操作:");
System.out.println("1、查询所有图书");
System.out.println("2、添加图书");
System.out.println("3、修改图书");
System.out.println("4、删除图书");
System.out.println("5、退出系统");
int choice = scanner.nextInt();
switch (choice) {
case 1:
List<Book> books = bookDao.getAllBooks();
for (Book book : books) {
System.out.println(book.getId() + "\t" + book.getName() + "\t" + book.getAuthor() + "\t" + book.getPrice());
}
break;
case 2:
System.out.println("请输入图书名称:");
String name = scanner.next();
System.out.println("请输入图书作者:");
String author = scanner.next();
System.out.println("请输入图书价格:");
double price = scanner.nextDouble();
Book book = new Book(0, name, author, price);
bookDao.addBook(book);
System.out.println("添加成功!");
break;
case 3:
System.out.println("请输入要修改的图书ID:");
int id1 = scanner.nextInt();
System.out.println("请输入图书名称:");
String name1 = scanner.next();
System.out.println("请输入图书作者:");
String author1 = scanner.next();
System.out.println("请输入图书价格:");
double price1 = scanner.nextDouble();
Book book1 = new Book(id1, name1, author1, price1);
bookDao.updateBook(book1);
System.out.println("修改成功!");
break;
case 4:
System.out.println("请输入要删除的图书ID:");
int id2 = scanner.nextInt();
bookDao.deleteBook(id2);
System.out.println("删除成功!");
break;
case 5:
System.exit(0);
default:
System.out.println("输入有误,请重新输入!");
break;
}
}
}
}
```
以上是一个简单的图书管理系统的示例代码,使用Java语言,使用IDEA开发工具和MySQL数据库。您可以根据自己的需求进行修改和定制。
阅读全文
相关推荐
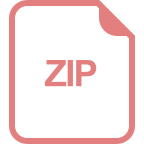

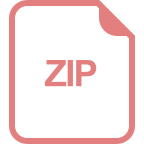
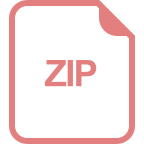
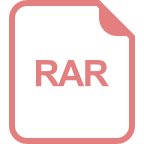
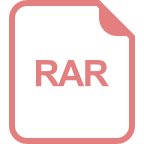
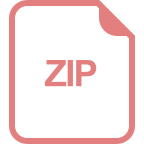
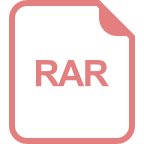
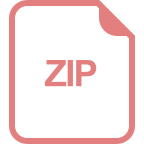
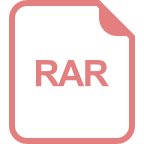
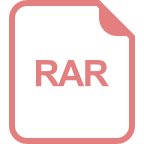
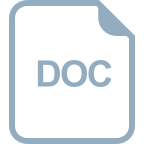
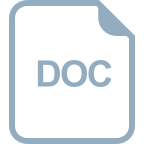
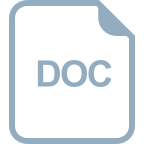
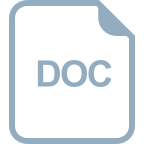

