C语言编程输入6个整数,将它们存入数组a中,再输入一个整数x,然后在数组中查找x,如果找到,输出相应的下标,否则,输出“Not Found”。
时间: 2024-05-07 18:16:29 浏览: 76
可以,这是一个常见的C语言编程问题。以下是代码示例:
```
#include <stdio.h>
#define SIZE 6
int main() {
int a[SIZE];
int x, i;
int found = 0;
// 读入数组a
for(i = 0; i < SIZE; i++) {
scanf("%d", &a[i]);
}
// 读入x
scanf("%d", &x);
// 在数组中查找x
for(i = 0; i < SIZE; i++) {
if(a[i] == x) {
found = 1;
printf("%d\n", i);
break;
}
}
// 输出结果
if(!found) {
printf("Not Found\n");
}
return 0;
}
```
希望这个代码示例可以帮助您!
相关问题
c语言 输入5个整数将它们存入数组排序再输入一个整数 然后在数组中查找这个数 统计要查找的数在数组中出现的次数
在C语言中,你可以通过以下几个步骤来实现这个功能:
1. 定义一个数组来存储五个整数,并提示用户输入这五个数。
```c
#include <stdio.h>
int main() {
int arr[5], i;
printf("请输入5个整数:");
```
2. 使用循环结构读取用户的输入并存入数组,记得验证输入的有效性。
```c
for (i = 0; i < 5; ++i) {
scanf("%d", &arr[i]);
}
```
3. 对数组进行排序,这里可以使用快速排序、冒泡排序或其他合适的算法,例如插入排序。
```c
// 排序部分,这里以冒泡排序为例
for (i = 0; i < 4; ++i) {
for (int j = 0; j < 4 - i; ++j) {
if (arr[j] > arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
```
4. 提示用户输入要查找的整数,并检查它是否在数组中。
```c
int search_num, count = 0;
scanf("%d", &search_num);
for (i = 0; i < 5; ++i) {
if (arr[i] == search_num) {
count++;
}
}
```
5. 输出查找结果,即该数字在数组中的出现次数。
```c
printf("数字 %d 在数组中出现了 %d 次。\n", search_num, count);
return 0;
}
```
c语言编程实现,输入10个互异的整数存入数组a中,再输入一个整数x,在数组a中利用二分法查找,若找到输出该数及在数组中的位置,若没有找到,将小于该数的所有数存入数组b中并输出。
您好!感谢您使用ChitGPT与我进行对话,我将为您解答问题。
以下是c语言实现的代码,实现了输入10个互异的整数存入数组a中,再输入一个整数x,在数组a中利用二分法查找,若找到输出该数及在数组中的位置,若没有找到,将小于该数的所有数存入数组b中并输出:
```
#include <stdio.h>
#define N 10
int a[N], b[N];
int main() {
int i, x, mid, left = 0, right = N - 1, flag = 0, cnt = 0;
printf("Please input the array a:\n");
for (i=0; i<N; i++) {
scanf("%d", &a[i]);
}
printf("Please input the integer x:\n");
scanf("%d", &x);
while (left <= right) {
mid = (left+right) / 2;
if (a[mid] == x) {
printf("Found %d at index %d.\n", x, mid);
flag = 1;
break;
} else if (a[mid] > x) {
right = mid - 1;
} else {
left = mid + 1;
}
}
if (!flag) {
printf("Did not find %d in array a.\n", x);
for (i=0; i<N; i++) {
if (a[i] < x) {
b[cnt++] = a[i];
}
}
printf("The integers smaller than %d in array a are:\n", x);
for (i=0; i<cnt; i++) {
printf("%d ", b[i]);
}
printf("\n");
}
return 0;
}
```
希望我的回答能够帮助到您,如有其他问题,请继续询问。
阅读全文
相关推荐
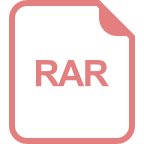
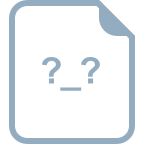
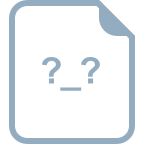













