基于stm32f103系列驱动RTC实时时钟并使用OLED显示的示例代码
时间: 2024-01-12 13:04:47 浏览: 27
好的,以下是基于STM32F103系列驱动RTC实时时钟并使用OLED显示的示例代码:
```c
#include "stm32f10x.h"
#include "stm32f10x_rcc.h"
#include "stm32f10x_rtc.h"
#include "stm32f10x_i2c.h"
#include "oled.h"
void RTC_Configuration(void);
void I2C_Configuration(void);
void OLED_Configuration(void);
void OLED_UpdateTime(RTC_TimeTypeDef RTC_TimeStruct, RTC_DateTypeDef RTC_DateStruct);
int main(void)
{
RTC_Configuration(); //初始化RTC
I2C_Configuration(); //初始化I2C
OLED_Configuration(); //初始化OLED
while(1)
{
RTC_TimeTypeDef RTC_TimeStruct;
RTC_DateTypeDef RTC_DateStruct;
RTC_GetTime(RTC_Format_BIN, &RTC_TimeStruct); //获取当前时间
RTC_GetDate(RTC_Format_BIN, &RTC_DateStruct); //获取当前日期
OLED_UpdateTime(RTC_TimeStruct, RTC_DateStruct); //更新OLED显示时间
}
}
void RTC_Configuration(void)
{
RCC_APB1PeriphClockCmd(RCC_APB1Periph_PWR | RCC_APB1Periph_BKP, ENABLE); //使能PWR和BKP外设时钟
PWR_BackupAccessCmd(ENABLE); //使能RTC和后备寄存器访问
RCC_LSEConfig(RCC_LSE_ON); //打开LSE外部晶振
while(RCC_GetFlagStatus(RCC_FLAG_LSERDY) == RESET); //等待LSE晶振稳定
RCC_RTCCLKConfig(RCC_RTCCLKSource_LSE); //将RTC时钟源改为LSE外部晶振
RCC_RTCCLKCmd(ENABLE); //使能RTC时钟
RTC_WaitForSynchro(); //等待RTC寄存器同步
RTC_InitTypeDef RTC_InitStructure;
RTC_InitStructure.RTC_HourFormat = RTC_HourFormat_24; //时间格式为24小时制
RTC_InitStructure.RTC_AsynchPrediv = 0x7F; //RTC异步分频系数为0x7F+1=128
RTC_InitStructure.RTC_SynchPrediv = 0xFF; //RTC同步分频系数为0xFF+1=256
RTC_Init(&RTC_InitStructure); //初始化RTC
RTC_TimeTypeDef RTC_TimeStruct;
RTC_TimeStruct.RTC_Hours = 0x00; //设置RTC小时数
RTC_TimeStruct.RTC_Minutes = 0x00; //设置RTC分钟数
RTC_TimeStruct.RTC_Seconds = 0x00; //设置RTC秒数
RTC_SetTime(RTC_Format_BIN, &RTC_TimeStruct); //设置RTC时间
RTC_DateTypeDef RTC_DateStruct;
RTC_DateStruct.RTC_Year = 0x20; //设置RTC年份
RTC_DateStruct.RTC_Month = RTC_Month_January; //设置RTC月份
RTC_DateStruct.RTC_Date = 0x01; //设置RTC日数
RTC_DateStruct.RTC_WeekDay = RTC_Weekday_Thursday; //设置RTC星期几
RTC_SetDate(RTC_Format_BIN, &RTC_DateStruct); //设置RTC日期
}
void I2C_Configuration(void)
{
RCC_APB1PeriphClockCmd(RCC_APB1Periph_I2C1, ENABLE); //使能I2C1外设时钟
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_6 | GPIO_Pin_7; //PB6和PB7引脚
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_OD; //开漏输出
GPIO_Init(GPIOB, &GPIO_InitStructure); //初始化GPIOB
I2C_InitTypeDef I2C_InitStructure;
I2C_InitStructure.I2C_Mode = I2C_Mode_I2C; //I2C模式
I2C_InitStructure.I2C_DutyCycle = I2C_DutyCycle_2; //占空比为2
I2C_InitStructure.I2C_OwnAddress1 = 0x00; //本地地址为0
I2C_InitStructure.I2C_Ack = I2C_Ack_Enable; //使能应答
I2C_InitStructure.I2C_AcknowledgedAddress = I2C_AcknowledgedAddress_7bit; //7位地址模式
I2C_InitStructure.I2C_ClockSpeed = 100000; //时钟速度为100kHz
I2C_Cmd(I2C1, ENABLE); //使能I2C1
I2C_Init(I2C1, &I2C_InitStructure); //初始化I2C1
}
void OLED_Configuration(void)
{
OLED_Init(); //初始化OLED
OLED_Clear(); //清屏
}
void OLED_UpdateTime(RTC_TimeTypeDef RTC_TimeStruct, RTC_DateTypeDef RTC_DateStruct)
{
char time_str[20];
sprintf(time_str, "%02d:%02d:%02d", RTC_TimeStruct.RTC_Hours, RTC_TimeStruct.RTC_Minutes, RTC_TimeStruct.RTC_Seconds);
char date_str[20];
sprintf(date_str, "%04d-%02d-%02d", RTC_DateStruct.RTC_Year, RTC_DateStruct.RTC_Month, RTC_DateStruct.RTC_Date);
OLED_ShowString(0, 0, "Time:");
OLED_ShowString(48, 0, time_str);
OLED_ShowString(0, 2, "Date:");
OLED_ShowString(48, 2, date_str);
}
```
这段代码中,我们在`main()`函数中调用`RTC_Configuration()`函数、`I2C_Configuration()`函数和`OLED_Configuration()`函数,分别初始化RTC、I2C和OLED。然后我们在一个无限循环中不断获取当前的时间和日期,并调用`OLED_UpdateTime()`函数来更新OLED上的时间和日期显示。`OLED_UpdateTime()`函数中,我们首先使用`sprintf()`函数将当前时间和日期转换为字符串。然后我们调用`OLED_ShowString()`函数来在OLED上显示时间和日期。
相关推荐
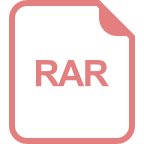
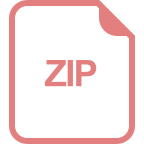














