使用c++实现学生管理系统并连接数据库存储数据实现代码
时间: 2024-03-03 12:54:00 浏览: 98
很抱歉,由于篇幅限制,不能在这里提供完整的代码。但是,我可以为您提供一个简单的示例,帮助您了解如何使用C++连接数据库并实现基本操作。以下是一个使用MySQL数据库和MySQL Connector/C++库的示例:
```c++
#include <iostream>
#include <mysql_connection.h>
#include <mysql_driver.h>
#include <cppconn/exception.h>
#include <cppconn/prepared_statement.h>
#include <cppconn/resultset.h>
using namespace std;
using namespace sql;
int main() {
try {
// 连接数据库
sql::Driver *driver;
sql::Connection *con;
driver = get_driver_instance();
con = driver->connect("tcp://localhost:3306", "root", "password");
con->setSchema("database_name");
// 插入学生信息
sql::PreparedStatement *pstmt;
pstmt = con->prepareStatement("INSERT INTO students (name, age, gender) VALUES (?, ?, ?)");
pstmt->setString(1, "张三");
pstmt->setInt(2, 18);
pstmt->setString(3, "男");
pstmt->executeUpdate();
// 查询学生信息
sql::Statement *stmt;
sql::ResultSet *res;
stmt = con->createStatement();
res = stmt->executeQuery("SELECT * FROM students");
while (res->next()) {
cout << res->getString("name") << "\t" << res->getInt("age") << "\t" << res->getString("gender") << endl;
}
// 删除学生信息
pstmt = con->prepareStatement("DELETE FROM students WHERE name = ?");
pstmt->setString(1, "张三");
pstmt->executeUpdate();
// 关闭连接
delete res;
delete stmt;
delete pstmt;
delete con;
} catch (sql::SQLException &e) {
cout << "SQLException: " << e.what() << endl;
}
return 0;
}
```
这个示例中,我们首先使用MySQL Connector/C++库连接到MySQL数据库,并指定要操作的数据库名称。然后,我们使用PreparedStatement对象向数据库中插入学生信息,使用Statement对象查询学生信息,以及使用PreparedStatement对象删除学生信息。最后,我们关闭连接并捕获任何可能发生的异常。
需要注意的是,这只是一个简单的示例,实际应用中还需要进行更多的错误处理和数据校验,以确保程序的稳定性和可靠性。
阅读全文
相关推荐
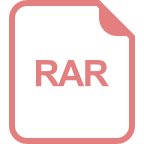
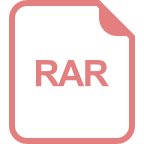
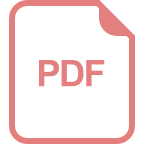
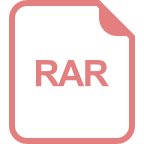
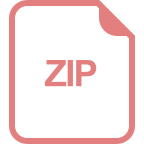
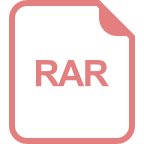
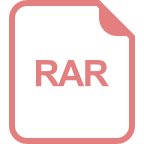
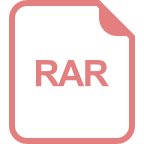
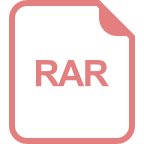
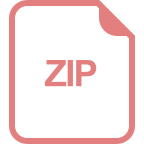
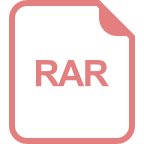
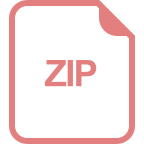
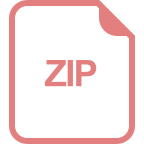
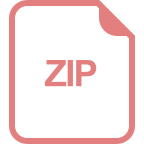
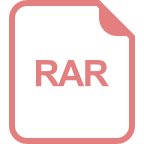
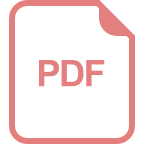
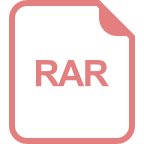
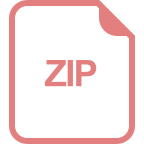