案例3-3:有两款配置和功能都不同的手机, 配置信息包括品牌,型号,操作系统,价格和内存; 手机功能包括自动拨号,游戏和播放歌曲。 本案例要求使用所学知识编写一个程序, 实现查看手机配置及功能,并将查看结果打印到控制台。用JAVA语言
时间: 2024-03-07 20:46:40 浏览: 61
好的,我可以为您提供一个简单的实现思路。
首先,我们需要定义一个手机类(Phone),包含以下属性:
- 品牌(brand)
- 型号(model)
- 操作系统(os)
- 价格(price)
- 内存(memory)
并且需要实现以下方法:
- 自动拨号(autoDial)
- 游戏(playGame)
- 播放歌曲(playMusic)
在实现上述方法时,可以直接在控制台输出相应的提示信息。具体实现可以参考以下代码:
```java
public class Phone {
private String brand;
private String model;
private String os;
private double price;
private int memory;
public Phone(String brand, String model, String os, double price, int memory) {
this.brand = brand;
this.model = model;
this.os = os;
this.price = price;
this.memory = memory;
}
public void autoDial() {
System.out.println("自动拨号功能正在开发中...");
}
public void playGame() {
System.out.println("游戏功能正在开发中...");
}
public void playMusic() {
System.out.println("播放歌曲功能正在开发中...");
}
public void showConfig() {
System.out.println("品牌:" + this.brand);
System.out.println("型号:" + this.model);
System.out.println("操作系统:" + this.os);
System.out.println("价格:" + this.price);
System.out.println("内存:" + this.memory);
}
}
```
接下来,我们可以创建一个测试类(Test),在其中创建两款手机并进行操作。具体实现可以参考以下代码:
```java
public class Test {
public static void main(String[] args) {
Phone phone1 = new Phone("Apple", "iPhone 12", "iOS", 6999, 128);
Phone phone2 = new Phone("Samsung", "Galaxy S21", "Android", 5999, 256);
System.out.println("手机1的配置:");
phone1.showConfig();
System.out.println("---------------------------------");
System.out.println("手机2的配置:");
phone2.showConfig();
System.out.println("---------------------------------");
System.out.println("手机1的功能:");
phone1.autoDial();
phone1.playGame();
phone1.playMusic();
System.out.println("---------------------------------");
System.out.println("手机2的功能:");
phone2.autoDial();
phone2.playGame();
phone2.playMusic();
}
}
```
当我们运行测试类时,控制台将输出以下结果:
```
手机1的配置:
品牌:Apple
型号:iPhone 12
操作系统:iOS
价格:6999.0
内存:128
---------------------------------
手机2的配置:
品牌:Samsung
型号:Galaxy S21
操作系统:Android
价格:5999.0
内存:256
---------------------------------
手机1的功能:
自动拨号功能正在开发中...
游戏功能正在开发中...
播放歌曲功能正在开发中...
---------------------------------
手机2的功能:
自动拨号功能正在开发中...
游戏功能正在开发中...
播放歌曲功能正在开发中...
```
这就完成了查看手机配置及功能的简单实现。
相关推荐
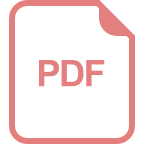
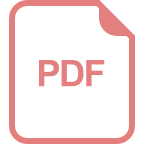
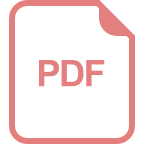














