MapReduce是使用Java编程吗
时间: 2024-05-29 16:15:50 浏览: 64
MapReduce是一个编程模型,可以使用多种编程语言实现,其中包括Java。实际上,Hadoop是一个使用Java编写的MapReduce框架,因此大多数使用Hadoop的MapReduce应用程序也是使用Java编写的。但是,也可以使用其他编程语言(如Python和C++)实现MapReduce程序。
相关问题
mapreduce的底层Java是怎样实现的
MapReduce的底层Java实现主要是通过使用Java编程语言和相关的类库来实现的。以下是MapReduce的基本实现步骤:
1.阶段:在该阶段输入数据被分为多个小块每个小块由一个Mapper任务处理。任务将输入数据映为键值对,并对每个键值对执行特定的操作。Mapper任务可以由Java中的Mapper类来实现,该类需要实现Mapper接口,并重写map()方法。
2. Shuffle阶段:在该阶段,Map产生的中间键值对会按照特定规则进行排序,并分发到不同的Reducer任务上。这个过程称为Shuffle。Shuffle的实现主要依赖于Java中的Partitioner、SortComparator和GroupComparator等类。
3. Reduce阶段:在该阶段,Reducer任务将Shuffle阶段输出的键值对进行聚合和处理。Reducer任务会将具有相同键的键值对组合在一起,并对它们执行特定的操作。Reducer任务可以由Java中的Reducer类来实现,该类需要实现Reducer接口,并重写reduce()方法。
在底层的实现中,还会涉及到一些其他的类和接口,比如InputFormat、OutputFormat、Job等,它们用于配置MapReduce任务的输入输出格式、作业控制等。
总结起来,MapReduce的底层Java实现是通过使用Java编程语言和相关的类库,结合各个阶段的接口和类来实现的。开发者可以根据自己的需求,使用Java语言来编写自定义的Mapper和Reducer类,以及其他相关的类和接口来实现MapReduce任务。
java使用mapreduce统计数据
在Java中使用MapReduce统计数据可以借助Hadoop框架来实现。下面是一个简单的示例代码,演示了如何使用MapReduce来统计数据:
首先,定义Mapper类,它会将输入数据分割为键值对并进行初步处理:
```java
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.LongWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Mapper;
public class MyMapper extends Mapper<LongWritable, Text, Text, IntWritable> {
private final static IntWritable one = new IntWritable(1);
private Text word = new Text();
public void map(LongWritable key, Text value, Context context)
throws IOException, InterruptedException {
String line = value.toString();
String[] words = line.split(" "); // 根据空格分割单词
for (String word : words) {
this.word.set(word);
context.write(this.word, one);
}
}
}
```
然后,定义Reducer类,它会对Mapper输出的键值对进行汇总处理:
```java
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Reducer;
public class MyReducer extends Reducer<Text, IntWritable, Text, IntWritable> {
public void reduce(Text key, Iterable<IntWritable> values, Context context)
throws IOException, InterruptedException {
int sum = 0;
for (IntWritable value : values) {
sum += value.get();
}
context.write(key, new IntWritable(sum));
}
}
```
最后,编写主程序来配置和运行MapReduce作业:
```java
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
public class WordCount {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "word count");
job.setJarByClass(WordCount.class);
job.setMapperClass(MyMapper.class);
job.setCombinerClass(MyReducer.class);
job.setReducerClass(MyReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
以上代码是一个简单的WordCount示例,它会统计输入文件中每个单词的出现次数。你可以根据具体需求修改Mapper和Reducer的实现逻辑,以实现其他类型的数据统计。
相关推荐
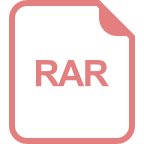
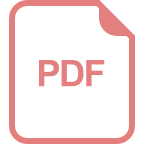
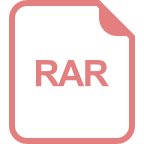
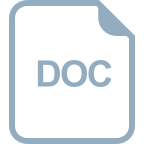
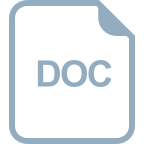
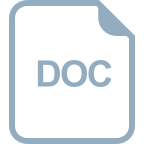
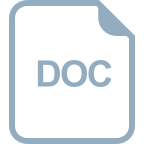
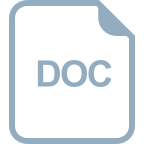







