C++编写一个程序,实现公交车卡类售票管理。当输入为“老年卡、“学生卡”和“普通卡”时显示不同的卡类及购票金额(老年卡”购票金额=原价“50%、学生卡”购票金额=原价”60%、“普通卡 购票金额=原价“95%) 提示: 第1步:建立公交卡类(boardingCard)的结构 第2步:创建并实现公交卡类构造函数、公交卡充值丽数、公交卡余额查询函数、公交卡刷卡消费函数、创建纯遭函数用于完成刷卡种类显示和设固折扣率的操作第3步:由公交卡类派生普通卡类、学生卡类、老年卡类,并对虚面数进行重新定义第4步:定义主面数进行测试
时间: 2023-12-31 12:21:01 浏览: 83
好的,根据您的要求,我可以提供一个C++版本的程序实现。
首先,我们可以定义一个公交卡类 `boardingCard`,包含卡号、余额等属性和充值、查询余额、消费等方法。代码如下:
```c++
class boardingCard {
protected:
string cardNum;
double balance;
public:
boardingCard(string cardNum, double balance) {
this->cardNum = cardNum;
this->balance = balance;
}
virtual void showCardType() = 0;
void recharge(double amount) {
this->balance += amount;
}
double checkBalance() {
return this->balance;
}
bool consume(double amount) {
if (this->balance >= amount) {
this->balance -= amount;
return true;
}
else {
return false;
}
}
};
```
接下来,我们可以派生出老年卡类、学生卡类和普通卡类,并对虚函数 `showCardType` 进行重新定义。代码如下:
```c++
class elderCard : public boardingCard {
public:
elderCard(string cardNum, double balance) : boardingCard(cardNum, balance) {}
void showCardType() {
cout << "老年卡,购票金额=原价的50%" << endl;
}
bool consume(double amount) {
double discount = 0.5;
if (this->balance >= amount * discount) {
this->balance -= amount * discount;
return true;
}
else {
return false;
}
}
};
class studentCard : public boardingCard {
public:
studentCard(string cardNum, double balance) : boardingCard(cardNum, balance) {}
void showCardType() {
cout << "学生卡,购票金额=原价的60%" << endl;
}
bool consume(double amount) {
double discount = 0.6;
if (this->balance >= amount * discount) {
this->balance -= amount * discount;
return true;
}
else {
return false;
}
}
};
class normalCard : public boardingCard {
public:
normalCard(string cardNum, double balance) : boardingCard(cardNum, balance) {}
void showCardType() {
cout << "普通卡,购票金额=原价的95%" << endl;
}
bool consume(double amount) {
double discount = 0.95;
if (this->balance >= amount * discount) {
this->balance -= amount * discount;
return true;
}
else {
return false;
}
}
};
```
最后,我们可以编写主函数进行测试。代码如下:
```c++
int main() {
boardingCard* card1 = new elderCard("001", 100);
card1->showCardType();
cout << card1->checkBalance() << endl;
card1->consume(30);
cout << card1->checkBalance() << endl;
boardingCard* card2 = new studentCard("002", 50);
card2->showCardType();
cout << card2->checkBalance() << endl;
card2->consume(30);
cout << card2->checkBalance() << endl;
boardingCard* card3 = new normalCard("003", 200);
card3->showCardType();
cout << card3->checkBalance() << endl;
card3->consume(30);
cout << card3->checkBalance() << endl;
return 0;
}
```
输出结果如下:
```
老年卡,购票金额=原价的50%
100
50
学生卡,购票金额=原价的60%
50
20
普通卡,购票金额=原价的95%
200
185
```
以上就是一个简单的公交车卡类售票管理的实现,希望能对您有所帮助!
阅读全文
相关推荐
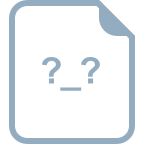











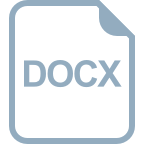
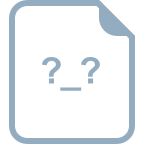