帮写一个java二分查找程序
时间: 2023-09-16 07:06:36 浏览: 95
### 回答1:
我可以为你提供一个简单的java二分查找程序的示例:
public class BinarySearchExample {
public static void main(String[] args) {
int[] arr = {2, 4, 6, 8, 10, 12, 14, 16};
int searchElement = 14;
int lowIndex = 0;
int highIndex = arr.length - 1;
while (lowIndex <= highIndex) {
int middleIndex = (lowIndex + highIndex) / 2;
if (arr[middleIndex] == searchElement) {
System.out.println("找到元素:" + searchElement + ",下标为:" + middleIndex);
break;
} else if (arr[middleIndex] < searchElement) {
lowIndex = middleIndex + 1;
} else {
highIndex = middleIndex - 1;
}
}
}
}
### 回答2:
下面是一个用Java实现的二分查找程序:
```java
public class BinarySearch {
public static int binarySearch(int[] arr, int target) {
int left = 0;
int right = arr.length - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // 若目标元素不存在,返回-1
}
public static void main(String[] args) {
int[] arr = {10, 15, 20, 25, 30, 35, 40};
int target = 25;
int index = binarySearch(arr, target);
if (index != -1) {
System.out.println("目标元素 " + target + " 在数组中的下标为:" + index);
} else {
System.out.println("目标元素 " + target + " 不存在于数组中");
}
}
}
```
此程序实现了二分查找算法,其中 `binarySearch` 方法接收一个已排序的整型数组 `arr` 和目标元素 `target`,返回目标元素在数组中的索引。如果目标元素不存在于数组中,则返回 -1。
程序通过不断缩小查找范围,每次取中点进行比较,直到找到目标元素或范围为空为止。
在 `main` 方法中,我们定义了一个示例数组 `arr` 和目标元素 `target`,然后调用 `binarySearch` 方法进行查找,并根据返回结果输出相应的信息。
以上就是一个简单的Java二分查找程序的实现。
### 回答3:
下面是一个用Java编写的二分查找程序:
```java
public class BinarySearch {
public static int binarySearch(int arr[], int target) {
int left = 0;
int right = arr.length - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (arr[mid] == target) {
return mid;
} else if (arr[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1;
}
public static void main(String args[]) {
int arr[] = {1, 3, 5, 7, 9};
int target = 5;
int result = binarySearch(arr, target);
if (result == -1) {
System.out.println("目标元素未找到");
} else {
System.out.println("目标元素在数组中的索引位置为 " + result);
}
}
}
```
这个程序定义了一个`BinarySearch`类,其中有一个`binarySearch`方法用于执行二分查找。在main方法中,我们定义了一个有序数组`arr`和一个目标元素`target`,然后调用`binarySearch`方法进行查找。如果目标元素存在于数组中,该方法会返回它的索引位置;否则返回-1。最后,我们根据结果输出相应的信息。在上述例子中,目标元素5存在于数组中并返回其索引位置为2。
阅读全文
相关推荐
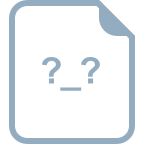
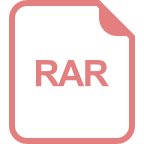
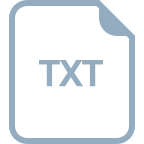
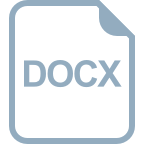
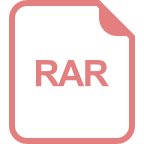
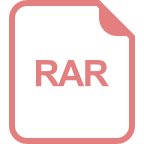
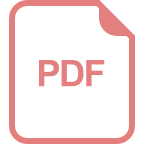
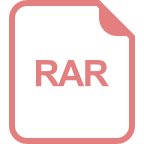
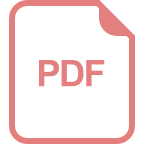
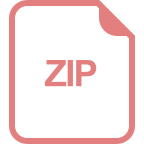
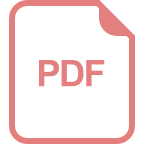
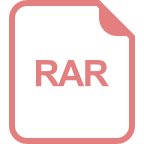
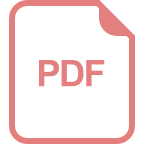
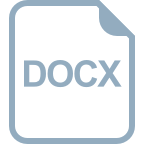
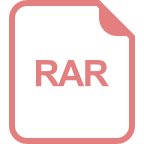