springboot高校工作室管理系统演示的代码
时间: 2024-09-15 13:01:12 浏览: 74
Spring Boot 高校工作室管理系统是一个基于 Spring Boot 框架构建的应用,它通常会包含用户管理、课程管理、项目管理等模块,用于模拟高等教育机构的工作室管理场景。由于这是一个完整的应用示例,涉及到的具体代码可能包括:
1. **启动类**(如`Application.java`):通过@EnableAutoConfiguration启用自动配置,并设置主程序入口。
```java
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
```
2. **实体类**(如`Student.java`, `Course.java`):用于表示用户、课程等实体的数据模型。
```java
@Entity
public class Student {
@Id
private Long id;
private String name;
// getters and setters
}
```
3. **服务层**(如`StudentService.java`):处理业务逻辑的方法,如添加学生、查询学生信息。
```java
@Service
public class StudentService {
@Autowired
private StudentRepository studentRepository;
public void addStudent(Student student) {
studentRepository.save(student);
}
public List<Student> getAllStudents() {
return studentRepository.findAll();
}
}
```
4. **控制器层**(如`UserController.java`):接收前端请求,调用服务层的方法并返回响应。
```java
@RestController
@RequestMapping("/api/students")
public class UserController {
@Autowired
private StudentService studentService;
@PostMapping
public ResponseEntity<?> createStudent(@RequestBody Student student) {
studentService.addStudent(student);
return ResponseEntity.ok().build();
}
@GetMapping
public ResponseEntity<List<Student>> getAllStudents() {
return ResponseEntity.ok(studentService.getAllStudents());
}
}
```
这只是简化的例子,实际项目会有更复杂的权限控制、数据验证、数据库操作、分页等功能,以及前端页面的展示部分。具体的代码你可以从Spring Boot官方文档或在线教程找到实例参考。
阅读全文
相关推荐
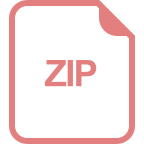
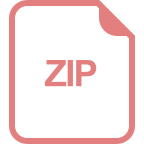
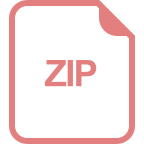
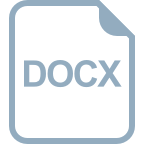
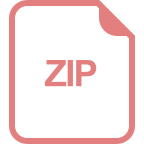
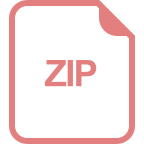
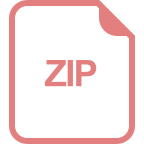
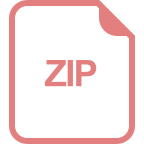
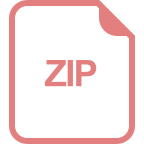
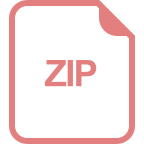
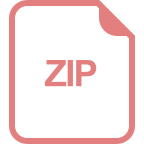
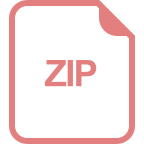
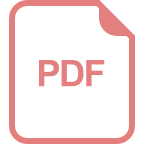